It looks like you're passing a vector of integers in the data parameter.
outliers <- (data[data < mean - cutoff * sd | data > mean + cutoff * sd])
.
With a silly example set a <- c(1, 2, 3, 4, 5, 6, 7, 8, 9)
this is searching for data < -3.215838 | data > 13.21584
which doesn't find a match.
I would default to using a package for outliers.
install.packages("outliers")
library(outliers)
values <- c(1, 1, 1, 8, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1)
outlier(values)
# prints [1] 8
Another option for time series data is Twitters package on anomaly detection
install.packages("devtools")
devtools::install_github("twitter/AnomalyDetection")
library(AnomalyDetection)
values <- c(1, 1, 1, 8, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1)
dates <- as.POSIXlt(c('2010-3-01', '2010-3-02','2010-3-03', '2010-3-04', '2010-3-05', '2010-3-06', '2010-3-07', '2010-3-08', '2010-3-09', '2010-3-10', '2010-3-11', '2010-3-12', '2010-3-13', '2010-3-14', '2010-3-15', '2010-3-16', '2010-3-17', '2010-3-18'
))
df <- data.frame(dates, values)
res = AnomalyDetectionTs(df, max_anoms=0.02, direction='both', plot=TRUE)
res$anoms
res$plot
# timestamp anoms
# 1 2010-03-04 8
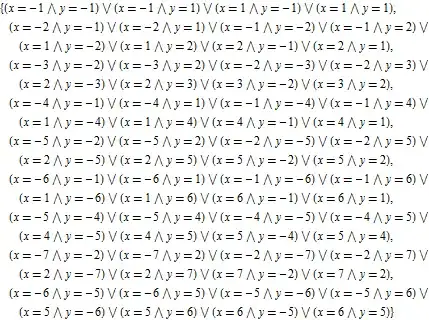