tl;dr
myPreparedStatement.setObject( … , LocalDate.parse( "2020-07-07" ) )
Date
class — not for dates
One of many flaws in the java.util.Date
class is its name. It does not represent a date. That class represents a moment, a date with time-of-day as seen in UTC.
Adding to the confusion is that its toString
method dynamically applies the JVM’s current default time zone.
Never use Date
Both the java.util.Date
class and java.sql.Date
class are terrible, flawed in design, written by people who did not understand date-time handling. Avoid them, along with SimpleDateFormat
etc. Sun, Oracle, and the JCP community gave up on those classes years ago, and so should you.
java.time
Those legacy classes were supplanted years ago by the modern java.time classes.
Your input string is in standard ISO 8601 format. The java.time classes use those formats by default when parsing/generating strings.
LocalDate ld = LocalDate.parse( "2020-07-07" ) ;
Database
You can exchange java.time object with your database as of JDBC 4.2 and later. Be sure your JDBC driver is up-to-date.
You should be writing a LocalDate
object to a column of a type akin to the SQL-standard type DATE
.
myPreparedStatement.setObject( … , ld ) ;
Retrieval.
LocalDate ld = myResultSet.getObject( … , LocalDate.class ) ;
About java.time
The java.time framework is built into Java 8 and later. These classes supplant the troublesome old legacy date-time classes such as java.util.Date
, Calendar
, & SimpleDateFormat
.
To learn more, see the Oracle Tutorial. And search Stack Overflow for many examples and explanations. Specification is JSR 310.
The Joda-Time project, now in maintenance mode, advises migration to the java.time classes.
You may exchange java.time objects directly with your database. Use a JDBC driver compliant with JDBC 4.2 or later. No need for strings, no need for java.sql.*
classes. Hibernate 5 & JPA 2.2 support java.time.
Where to obtain the java.time classes?
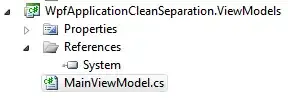