Here is a complete, working example using the Pokémon Stats data. We can automate the conversion of columns by obtaining the column types from the input data.
gen01file <- "https://raw.githubusercontent.com/lgreski/pokemonData/master/gen01.csv"
gen01 <- read.csv(gen01file,header=TRUE,stringsAsFactors = FALSE)
At this point, gen01
data frame consists of some character columns, some integer columns, and a logical column.

Next, we'll extract the column types with a combination of lapply()
and unlist()
.
# extract the column types
colTypes<- unlist(lapply(gen01[colnames(gen01)],typeof))
At this point, colTypes
is a vector that contains the column types, where each element is named by the column name. This is important, because now we can extract the names and automate the process of converting character variables to factor, and integer / double variables with a log10()
transformation.
# find character types to convert to factor, using element names from
# colTypes vector
factorColumns <- names(colTypes[colTypes == "character"])
logColumns <- names(colTypes[colTypes %in% c("integer","double")])
Note that at this point we could potentially subset the column name objects further (e.g. use regular expressions to pull certain names from the list of columns, given their data type).
Finally, we use lapply()
to apply the appropriate transform on the relevant columns, as noted in Ronak Shah's answer.
gen01[factorColumns] <- lapply(gen01[factorColumns],factor)
gen01[logColumns] <- lapply(gen01[logColumns],log10)
As we can see from the RStudio object viewer, the character variables are now factors, and the values of the integer columns have been log transformed. The Legendary
logical column is untouched.
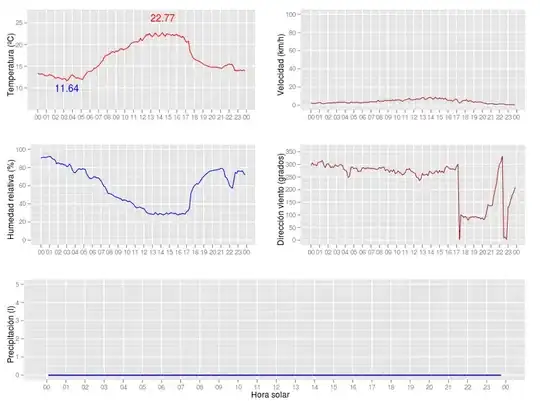