As others mentioned, you can use scale_color_gradientn()
to specify a gradient of n
number of colors and make your own gradient. You can check the documentation on the function for more information, but here's a working example.
library(ggplot2)
set.seed(1234)
df <- data.frame(
x=1:200,
y=rnorm(200, 1, 0.2),
cols=sample(1:10, 200, replace = TRUE)
)
p <- ggplot(df, aes(x,y, color=cols)) + geom_point()
p + scale_color_gradientn(colors=c('blue','green','yellow','red'))
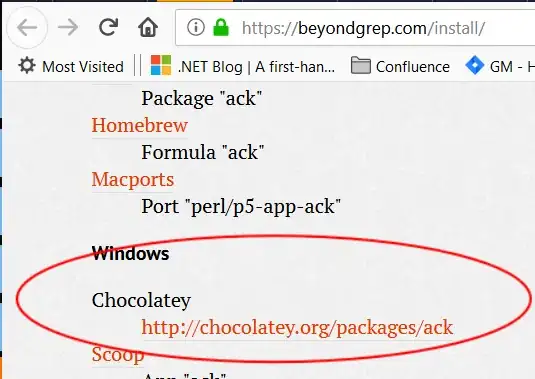
That looks great, but what if you wanted to (as you mentioned in your case), specify a particular position for the colors? In this case, let's say we wanted the df$cols==5.0
to be yellow.
You can do that by supplying a vector to the values=
argument. However, there is a bit of a trick to this that is not obvious from the documentation. The values=
argument expects a vector to indicate the positions of each of your colors=
on a scale from 0 to 1. Note how we have our df$cols
ranging from 0 to 10, so that means that df$cols==5.0
is represented by values=0.5
.
p + scale_color_gradientn(
colors=c('blue','green','yellow','red'),
values=c(0,0.2,0.5,1)
)
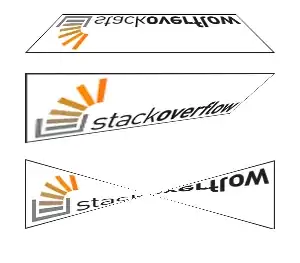