It sounds like a bug in the Street View Image API. If I check the pano id CAoSLEFGMVFpcFB3ZWxWT21OX196WVc2ajlGeE9qbGptTHFHc2RNRjBwRlYyamhw
from your example using the Street View Image Metadata API:
https://maps.googleapis.com/maps/api/streetview/metadata?pano=CAoSLEFGMVFpcFB3ZWxWT21OX196WVc2ajlGeE9qbGptTHFHc2RNRjBwRlYyamhw&key=MY_API_KEY
I get the following response
{
"copyright":"© Miller Yu",
"date":"2018-11",
"location":{
"lat":35.6894875,
"lng":139.6917064
},
"pano_id":"CAoSLEFGMVFpcFB3ZWxWT21OX196WVc2ajlGeE9qbGptTHFHc2RNRjBwRlYyamhw",
"status":"OK"
}
That means that pano id is valid, it exists in Google database, but it is not available via Street View Image API.
We can see that this pano was uploaded by external user, so it is not typical Google street view image. I know that Google experienced issues with business generated panos in the past. For reference you can have a look at the following bugs in the Google issue tracker:
https://issuetracker.google.com/issues/35829459
https://issuetracker.google.com/issues/77676413
Both are marked as fixed, however it looks like in your case it is not true. At this point I would suggest report an issue to Google via their issue tracker again.
As a workaround consider requesting only Google's outdoor imagery. You can specify source
property in your Street View pano request as shown in my example
function initialize() {
let svService = new google.maps.StreetViewService();
let tokyo = {lat: 35.6895,lng: 139.6917};
var panoRequest = {
location: tokyo,
preference: google.maps.StreetViewPreference.NEAREST,
radius: 250,
source: google.maps.StreetViewSource.OUTDOOR
};
svService.getPanorama(panoRequest, svCheck);
function svCheck(data, status) {
if (status === google.maps.StreetViewStatus.OK) {
console.log("OK for "+data.location.latLng+" at ID "+data.location.pano);
var panorama = new google.maps.StreetViewPanorama(
document.getElementById('street-view'),
{
pano: data.location.pano,
});
}
else {
console.log("STATUS NOT OK");
}
}
}
html, body {
height: 100%;
margin: 0;
padding: 0;
}
#street-view {
height: 100%;
}
<div id="street-view"></div>
<script async defer
src="https://maps.googleapis.com/maps/api/js?key=AIzaSyDztlrk_3CnzGHo7CFvLFqE_2bUKEq1JEU&callback=initialize">
</script>
With modified request you will get a pano ID rHMU4M8-OvVuL5ALS0OlNg
. If you check it with metadata API
https://maps.googleapis.com/maps/api/streetview/metadata?pano=rHMU4M8-OvVuL5ALS0OlNg&key=YOUR_API_KEY
you will get the following response
{
"copyright":"© Google",
"date":"2016-07",
"location":{
"lat":35.68943918433396,
"lng":139.6914496241509
},
"pano_id":"rHMU4M8-OvVuL5ALS0OlNg",
"status":"OK"
}
This pano belongs to Google and it works correctly with Street View Image API
https://maps.googleapis.com/maps/api/streetview?pano=rHMU4M8-OvVuL5ALS0OlNg&size=600x400&key=YOUR_API_KEY
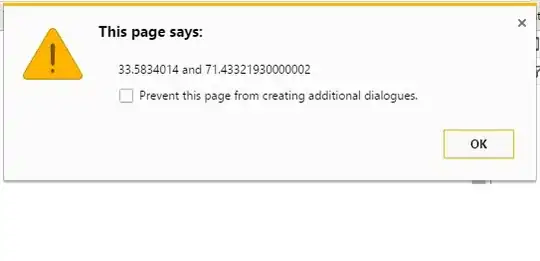