We can also use the channel factory to call WCF services, this method does not need to add a service reference,here is a demo:
BasicHttpBinding basicHttpBinding = new BasicHttpBinding();
var address = new EndpointAddress("http://localhost:801/Service1.svc/Service");
var factory = new ChannelFactory<IService1>(basicHttpBinding, address);
IService1 channel = factory.CreateChannel();
channel.GetData(1);
Console.WriteLine(channel.GetData(1));
Console.ReadLine();
On the client side, we need to have a ServiceContract:
[ServiceContract]
public interface IService1
{
[OperationContract]
string GetData(int value);
[OperationContract]
CompositeType GetDataUsingDataContract(CompositeType composite);
// TODO: Add your service operations here
}
This ServiceContract is the same as the ServiceContract on the server side.
Because you are calling WCF in core, you need to add the following two packages:

If you use NetTcpBinding, you need to add the following package:
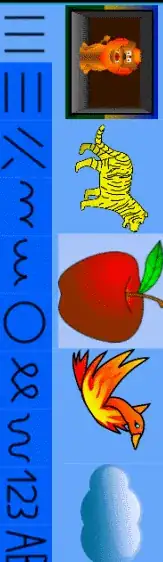
In addition, there are some limitations when calling WCF in core. You can refer to this link:
https://github.com/dotnet/wcf/blob/master/release-notes/SupportedFeatures-v2.1.0.md
Feel free to let me know if the problem persists.