I am also learning so in order to exercise a bit i have created a very simple example for you. It is probably unprofessional and unsafe but i think (hope) it is possible to extend it somehow :).
Firstly you need to create simple WPF windows (use txt/btn+Name naming convention):
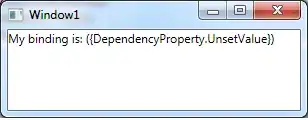
For both windows add
using System.IO;
Then you need to add events for buttons and modify code for both windows:
public partial class LoginWindow : Window
{
public LoginWindow()
{
InitializeComponent();
}
// This is really bad/weak encryption method
String WeakDecryptMethod(String textIn)
{
Char[] temp = textIn.ToArray<Char>();
for (int i = 0; i < textIn.Length; i++)
{
temp[i] = (char)((int)temp[i] - 3);
}
return new String(temp);
}
private void btnRegister_Click(object sender, RoutedEventArgs e)
{
RegisterWindow newWindow = new RegisterWindow();
newWindow.ShowDialog();
}
private void btnOK_Click(object sender, RoutedEventArgs e)
{
// If file exist and login and password are "correct"
if (File.Exists("Users.txt")
&& txtLogin.Text.Length >= 4
&& txtPass.Text.Length >= 4)
{
using (StreamReader streamReader = new StreamReader("Users.txt"))
{
// While there is something in streamReader read it
while (streamReader.Peek() >= 0)
{
String decryptedLogin = WeakDecryptMethod(streamReader.ReadLine());
String decryptedPass = WeakDecryptMethod(streamReader.ReadLine());
if (decryptedLogin == txtLogin.Text && decryptedPass == txtPass.Text)
{
ProtectedWindow protectedWindow = new ProtectedWindow();
this.Close();
protectedWindow.Show();
break;
}
}
}
}
}
private void btnCancel_Click(object sender, RoutedEventArgs e)
{
this.Close();
}
}
And code for Register window:
public partial class RegisterWindow : Window
{
public RegisterWindow()
{
InitializeComponent();
}
// This is really bad/weak method to encrypt files
String WeakEncryptMethod(String textIn)
{
Char[] temp = textIn.ToArray<Char>();
for (int i = 0; i < textIn.Length; i++)
{
temp[i] = (char)((int)temp[i] + 3);
}
return new String(temp);
}
private void btnRegister_Click(object sender, RoutedEventArgs e)
{
// If file exist and login and password are "correct"
if (File.Exists("Users.txt")
&& txtLogin.Text.Length >= 4
&& txtPass.Text.Length >= 4
&& txtPass.Text == txtPassCheck.Text)
{
StringBuilder stringBuilder = new StringBuilder();
using (StreamReader streamReader = new StreamReader("Users.txt"))
{
stringBuilder.Append(streamReader.ReadToEnd());
}
using (StreamWriter streamWriter = new StreamWriter("Users.txt"))
{
streamWriter.Write(stringBuilder.ToString());
streamWriter.WriteLine(WeakEncryptMethod(txtLogin.Text));
streamWriter.WriteLine(WeakEncryptMethod(txtPass.Text));
}
this.Close();
}
}
private void btnCancel_Click(object sender, RoutedEventArgs e)
{
this.Close();
}
}
In order to work application need to have access to file "Users.txt" which needs to be placed in the same folder.
Notes:
- It will be better if you will use some proper encryption functions and probably create separate class for it. Additionally i am almost sure that it will not work properly with login and password which contains the last 3 characters from the end of ASCII tables.
- In my opinion it is a bad idea to store login and password data in *.txt file :).
- As far i know C# code is very easily reverse engineered so probably it will be better to hide encryption/decryption part somehow. I do not know much about it, but u will be able to read more [here] 2 and probably uncle Google will be able to help.
- Code is very simple and there is probably a lot of possibilities to extend it (more file handling stuff, TextBox validation for proper input and password strength calculations)