For complex reference types that refer to other reference types, there is not a simple way to flatten those out into a pure sequence of bytes and there is a reason serializers have to use more complex formats to handle such cases.
That said, the type you show is what is called a blittable type.
Blittable types only contain fields of other primitive types whose bit pattern can be directly copied.
By changing your class to a struct, you could generically persist such types to disk directly. This does require the use of an unsafe block, but the code is relatively simple.
There are ways to do the same thing without using unsafe code, but using unsafe is the most straight-forward since you can just take a pointer and use that to write directly to disk, the network, etc.
First, change to a struct:
[StructLayout(LayoutKind.Sequential)]
public struct VarMap
{
public byte var1;
public long var2;
public int var3;
}
Then, create one and write it directly to disk:
VarMap vm = new VarMap
{
var1 = 254,
var2 = 1234,
var3 = 5678
};
unsafe
{
VarMap* p = &vm;
int sz = sizeof(VarMap);
using FileStream fs = new FileStream(@"out.bin", FileMode.Create);
ReadOnlySpan<byte> span = new ReadOnlySpan<byte>(p, sz);
fs.Write(span);
}
However, if you look at the result in a hex viewer you will notice something:
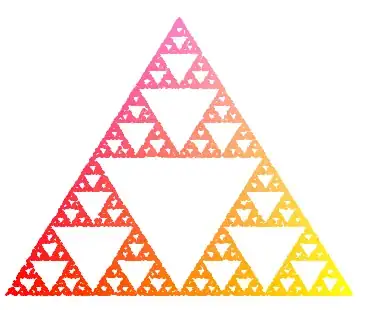
24 bytes were written to disk, not the 13 that we might assume based on the types of the fields in your data structure.
This is because structures are padded in memory for alignment purposes.
Depending on what the device you are sending these bytes to actually expects (and endian-ness might also be an issue), this kind of technique may or may not work.
If you need absolute control, you probably want to hand-write the serialization code yourself rather than trying to find some generic mechanism to do this. It might be possible using reflection, but probably simpler to just convert your fields to the raw byte representation directly.