Edit: Since the OP made edits to the question and the followup questions raised by others I have edited my answer.
To start with, List to LinkedList does not have any implicit or explicit conversion.
However, the following demo code compiles. While this is not exactly the same as the OP's stated question, I have broken down the steps.
using System;
using System.Linq;
using System.Collections.Generic;
using System.Data.Entity;
namespace linkedlist
{
class Program
{
static void Main(string[] args)
{
var arr = new [] { "first", "second", "third" };
var q1 = from x in arr
select x;
var q = q1.ToList();
var ll = new LinkedList<string>(q);
}
}
}
Why not work with the List directly, unless you are performing a lot of operations for which the LinkedList is optimal compared to List (See this SO query and answers on List vs LinkedList)?
The last statement creates a new LinkedList<T>
which copies the contents of the List<T>
.
If the intent is to obtain a LinkedList anyway from a LINQ query, the following is less wasteful:
static void Main(string[] args)
{
Console.WriteLine("Hello World!");
var arr = new [] { "first", "second", "third" };
var q1 = from x in arr
select x;
var ll = new LinkedList<string>(q1);
}
Experiments
Here is some data on performance of
Linq
to List<T>
to LinkedList<T>
vs. Linq
to LinkedList<T>
Experiment code
using System;
using System.Linq;
using System.Collections.Generic;
using System.Data.Entity;
namespace linkedlist
{
class Program
{
static void Main(string[] args)
{
var arr = new[] { "first", "second", "third" };
var q1 = from x in arr
select x;
for(var j = 0; j < 10; j++)
{
var st1 = DateTime.Now;
for (var i = 0; i < 10000; i++)
{
var q = q1.ToList();
var ll = new LinkedList<string>(q);
}
var en1 = DateTime.Now;
Console.WriteLine($"With LINQ to List<T> to LinkedList<T> {(en1 - st1).TotalMilliseconds}");
var st2 = DateTime.Now;
for (var i = 0; i < 10000; i++)
{
var ll = new LinkedList<string>(q1);
}
var en2 = DateTime.Now;
Console.WriteLine($"With LinQ to LinkedList<T> {(en2 - st2).TotalMilliseconds}");
}
}
}
}
Timing Results:
Screenshot:
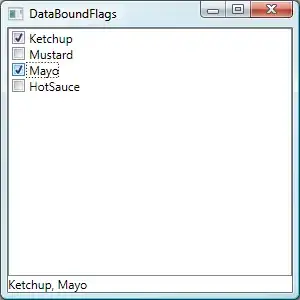
The first run should be ignored to discount cache warm-up effect as we are using the same LINQ query for both tests. All times are Total Elapsed Milliseconds for 10,000 iterations per trial and a total of 10 trials.
With LINQ to List<T> to LinkedList<T> 20.0769
With LinQ to LinkedList<T> 8.6571
With LINQ to List<T> to LinkedList<T> 12.0162
With LinQ to LinkedList<T> 8.7212
With LINQ to List<T> to LinkedList<T> 12.7354
With LinQ to LinkedList<T> 8.3412
With LINQ to List<T> to LinkedList<T> 12.6848
With LinQ to LinkedList<T> 8.1306
With LINQ to List<T> to LinkedList<T> 12.3656
With LinQ to LinkedList<T> 8.5935
With LINQ to List<T> to LinkedList<T> 19.8802
With LinQ to LinkedList<T> 7.9596
With LINQ to List<T> to LinkedList<T> 12.1177
With LinQ to LinkedList<T> 9.0604
With LINQ to List<T> to LinkedList<T> 18.219
With LinQ to LinkedList<T> 11.115
With LINQ to List<T> to LinkedList<T> 18.4309
With LinQ to LinkedList<T> 11.2717
With LINQ to List<T> to LinkedList<T> 18.6743
With LinQ to LinkedList<T> 11.4202
Conclusion:
Linq to LinkedList is faster than Linq to List to LinkedList and less wasteful.