Let me explain with Interactive console:
>>> original_list = [1, 2, 3, 4] # source
>>> reference = original_list # 'aliasing' to another name.
>>> reference is original_list # check if two are referencing same object.
True
>>> id(reference) # ID of referencing object
1520121182528
>>> id(original_list) # Same ID
1520121182528
To create new list:
>>> copied = list(original_list)
>>> copied is original_list # now referencing different object.
False
>>> id(copied) # now has different ID with original_list
1520121567616
There's multiple way of copying lists, for few examples:
>>> copied_slicing = original_list[::]
>>> id(copied_slicing)
1520121558016
>>> import copy
>>> copied_copy = copy.copy(original_list)
>>> id(copied_copy)
1520121545664
>>> copied_unpacking = [*original_list]
>>> id(copied_unpacking)
1520123822336
.. and so on.
Image from book 'Fluent Python' by Luciano Ramalho might help you understand what's going on:
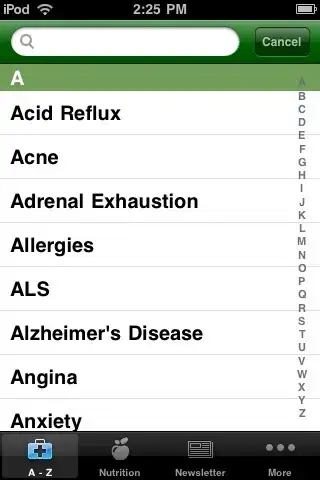
Rather than 'name' being a box that contains respective object, it's a Post-it stuck at object in-memory.