If you're talking about a temporary solution, for less than 1000 products, and its going to be once off, even if it's a once off per year, then it might be easier to tell your user to submit the excel file to you with their products, and then you can use direct DBA techniques to import this data into the database manually.
I feel that this post sums up your scenario: Time is running out...
The idea is to identify how long it takes you to complete the task (Import data from an Excel spreadsheet) and then to identify how many times you are likely to perform this task..
If user presents to you an Excel file of a flat data structure, with a bit of SQL Wizardry and Ninja skills, 10 minutes later you can have that data inserted into the database using SQL alone.
- This would be a good topic for a question to ask on this forum, try it out, then post a question when/if you get stuck.
- So if you are only going to do this task once or twice, ever... then don't take more than 10-20 minutes to develop a coded solution that the user can use.
- Even if it only took you 10 minutes, you could reasonably charge the client a couple of hours for the privilege, but the reverse is less reasonable, you could probably only charge for a few hours to code, deploy and teach the user to use this new functionality, even though that full process may take a few days of back and forth effort to get it right, I would consider it highway robbery to charge much for this sort of feature if they aroun only going to do it once.
Instead of SQL skills, you could still write your own C# script specifically to import the user's file, it doesn't have to be client facing, a simple c# script either as a standalone console app, unit test or a hidden method in your application runtime could be written in less than an hour, then it might take you 10 minutes to take the excel data and massage it so that your script can use it.
But the time it takes you, vs what you can reasonably charge the user for this functionlity must still be taken into account.
So once you've identified how long the task will take you, and how many times you will need to perform this task in the future, then you can follow tables like this to identify if it is worth the effort to automate the task at all:
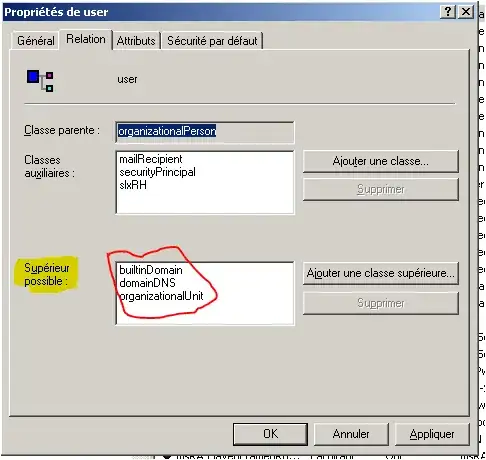
Originally from XKCD: Is It Worth the Time?
If you can write good solid client facing solution into your app where the client can select the file to upload and your code automates the rest, then you still only want this to take few hours at most, certainly possible, but unless you do it well, you will probably get higher customer satisfaction by saving them some $$$ and performing this task for them on demand, then offering them a buggy interface that they have to pay $1000s of dollars for.
Tasks like this are less about how to write the code to complete the task, and more about what is the right business decision for you and your client, which brings me to me second favorite sketch:
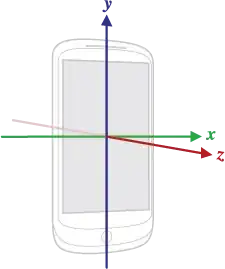
Originally from XKCD: Automation
So while you think you want to write code to allow your user to import data from excel for one time only... there might be a different way to solve your problem.