First of all, pointers of string type are not the same as pointers of char type. You can refer to the following code.
#include <iostream>
using namespace std;
int main(int argc, const char* argv[])
{
string* s = new string("Abc");
cout << s << endl;
cout << *s << endl;
return 0;
}
Output:
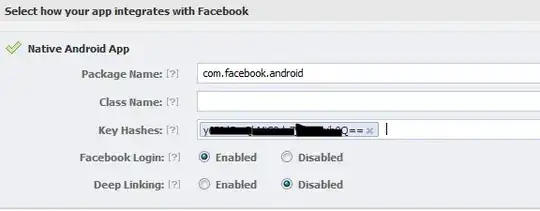
And *s
is the same as str[0]
, so the str_ptr[0] ='D'
you write will overwrite the original string.
Also, when you use str_ptr
directly, you are using its address instead of its elements, which is different from using:
char* str_ptr = str;
str_ptr[0] = 'D';
The string is different from the char type string in the C language. The string pointer points to a class object. If you want to modify the content of the string through the string pointer, you can try the following code:
string* str_ptr = &str;
str_ptr->at(0) = 'D';
And you cannot directly use str_ptr
as the parameter of %s
, it should be str_ptr->c_str()
.
Here is the complete sample:
#include <iostream>
#include <windows.h>
#include <WinUser.h>
using namespace std;
int main(int argc, const char* argv[])
{
string str = "Abc";
string* str_ptr = &str;
str_ptr->at(0) = 'D';
char MsgBuff[300];
sprintf_s(MsgBuff, 300, "\nMSG = %s\n", str_ptr->c_str());
OutputDebugStringA(MsgBuff);
return 0;
}
And it works for me:
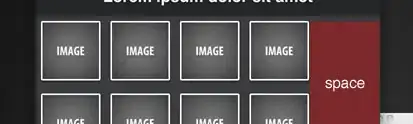