Article/Reference: Arrays in query params
Retrieve Option 1
var request = HttpContext.Current.Request;
string[] StorageAddress = request.Params["StorageAddress"].Split(',');
Retrieve Option 2
public IHttpActionResult Get([FromUri] string[] StorageAddress )
{
....
}
UPDATE with PostMan passing JSON
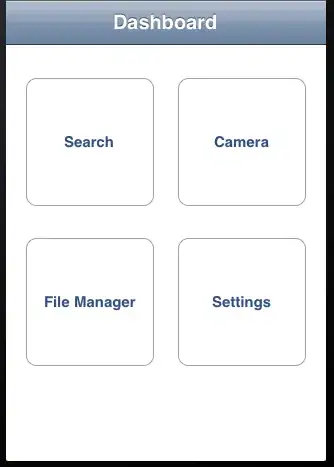
You can use Retrieve Option 3
public IHttpActionResult Get(string[] StorageAddress )
{
....
}
Retrieve Option 4 with Newtonsoft
var json = await Request.Content.ReadAsStringAsync();
var storageAddress = JsonConvert.DeserializeObject<string[]>(json);
Retrieve Option 5 for mix of json data and files. For postman passing you refer to this How to upload a file and JSON data in Postman?.
public async Task<IHttpActionResult> Post()
{
var atorageAddress = HttpContext.Current.Request.Form["StorageAddress"].Split(',');
var files = HttpContext.Current.Request.Files;
List<HttpPostedFile> fileList = new List<HttpPostedFile>();
foreach (var key in files.AllKeys)
{
// Do your logic here for manipulation of the files
fileList.Add(files[key]);
}
return Json("OK");
}
UPDATE: Retrieve JSON
Set your JSON like this.
[{"Address":"Test Store 1"},{"Address":"Test Store 2"}]
Retrieve it like this.
var storageAddress = JsonConvert.DeserializeObject<List<Dictionary<string, string>>>(HttpContext.Current.Request.Form["StorageAddress"]);