You are not converting the DateTime
object to java.util.Date
correctly. A correct way is to get the milliseconds from DateTime
object and initialize the java.util.Date
with the milliseconds.
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.TimeZone;
import org.joda.time.DateTime;
import org.joda.time.format.DateTimeFormat;
import org.joda.time.format.DateTimeFormatter;
public class Main {
public static void main(String[] args) {
// Define formatter
DateTimeFormatter formatter = DateTimeFormat.forPattern("EEE MMM dd HH:mm:ss zZ yyyy");
// Date-time string from DatePicker
String strDateTime = "Thu Sep 10 00:00:00 GMT+03:00 2020";
DateTime dateTime = DateTime.parse(strDateTime, formatter);
System.out.println(dateTime);
// No. of milliseconds from the epoch of 1970-01-01T00:00:00Z
long millis = dateTime.getMillis();
System.out.println(millis);
Date mDate = new Date(millis);
// Display java.util.Date object in my default time-zone (BST)
System.out.println(mDate);
//Display java.util.Date in a different time-zone and using custom format
SimpleDateFormat sdf = new SimpleDateFormat("EEE MMM dd HH:mm:ss z yyyy");
sdf.setTimeZone(TimeZone.getTimeZone("GMT+3"));
System.out.println(sdf.format(mDate));
}
}
Output:
2020-09-09T21:00:00.000Z
1599685200000
Wed Sep 09 22:00:00 BST 2020
Thu Sep 10 00:00:00 GMT+03:00 2020
Note: java.util.Date
does not represent a Date/Time object. It simply represents the no. of milliseconds from the epoch of 1970-01-01T00:00:00Z
. It does not have any time-zone or zone-offset information. When you print it, Java prints the string obtained by applying the time-zone of your JVM. If you want to print it in some other timezone, you can do so using the SimpleDateFormat
as shown above.
I recommend you switch from the outdated and error-prone java.util
date-time API and SimpleDateFormat
to the modern java.time
date-time API and the corresponding formatting API (package, java.time.format
). Learn more about the modern date-time API from Trail: Date Time. If your Android API level is still not compliant with Java8, check How to use ThreeTenABP in Android Project and Java 8+ APIs available through desugaring.
The following table shows an overview of modern date-time classes:
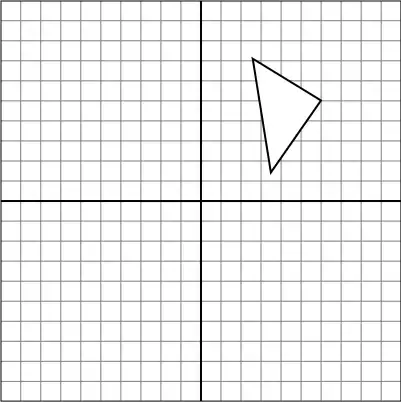