OP and Welcome to SO. In future questions, please, be sure to provide a minimal reproducible example - meaning provide code, an image (if possible), and at least a representative dataset that can demonstrate your question or problem clearly.
TL;DR - don't use stat="identity"
, just use geom_bar()
without providing a stat, since default is to use the counts. This should work:
ggplot(chart_data, aes(x = Amino.Acid, fill = tRF.type)) + geom_bar()
The dataset provided doesn't adequately demonstrate your issue, so here's one that can work. The example data herein consists of 100 observations and two columns: one called Capitals
for randomly-selected uppercase letters and one Lowercase
for randomly-selected lowercase letters.
library(ggplot2)
set.seed(1234)
df <- data.frame(
Capitals=sample(LETTERS, 100, replace=TRUE),
Lowercase=sample(letters, 100, replace=TRUE)
)
If I plot similar to your code, you can see the result:
ggplot(df, aes(x=Capitals, y=Lowercase, fill=Lowercase)) +
geom_bar(stat="identity")
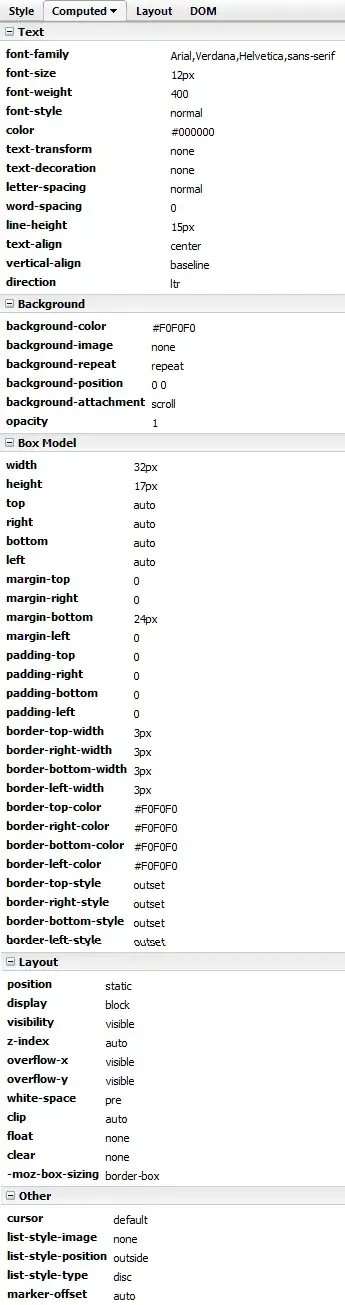
You can see, the bars are stacked, but the y axis is all smooshed down. The reason is related to understanding the difference between geom_bar()
and geom_col()
. Checking the documentation for these functions, you can see that the main difference is that geom_col()
will plot bars with heights equal to the y
aesthetic, whereas geom_bar()
plots by default according to stat="count"
. In fact, using geom_bar(stat="identity")
is really just a complicated way of saying geom_col()
.
Since your y
aesthetic is not numeric, ggplot
still tries to treat the discrete levels numerically. It doesn't really work out well, and it's the reason why your axis gets smooshed down like that. What you want, is geom_bar(stat="count")
.... which is the same as just using geom_bar()
without providing a stat=
.
The one problem is that geom_bar()
only accepts an x
or a y
aesthetic. This means you should only give it one of them. This fixes the issue and now you get the proper chart:
ggplot(df, aes(x=Capitals, fill=Lowercase)) + geom_bar()
