If you look at the response headers provided by the remoteUri, you will notice that the particular endpoint is actually serving content in compressed format.
Content-Encoding: gzip
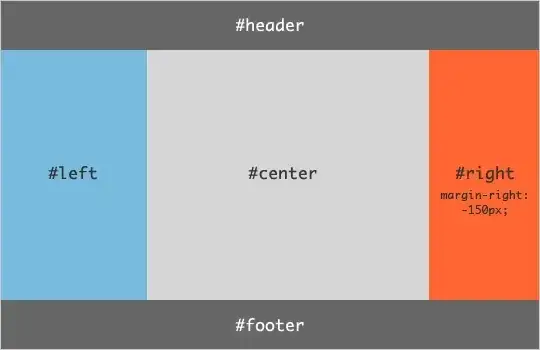
So the content you get back is not a direct excel file, rather a zip file. So for the piece of code to work, the file name should be temp.zip
instead of temp.xlsx
private void downloadFile()
{
string remoteUri = "http://members.tsetmc.com/tsev2/excel/MarketWatchPlus.aspx?d=0";
string fileName = @"g:\temp.zip";
using (var client = new WebClient())
{
client.DownloadFile(remoteUri, fileName);
}
}
Having said that, inline is a better approach to download the file.
Create an instance of HttpClient
by passing in a HttpClientHandler
which has the AutomaticDecompression
property set to DecompressionMethods.GZip
to handle Gzip decompression automatically. Next read the data and save it to temp.xlsx
file.
string remoteUri = "http://members.tsetmc.com/tsev2/excel/MarketWatchPlus.aspx?d=0";
string fileName = @"g:\temp.xlsx";
HttpClientHandler handler = new HttpClientHandler()
{
AutomaticDecompression = DecompressionMethods.GZip | DecompressionMethods.Deflate
};
HttpClient client = new HttpClient(handler);
var response = await client.GetAsync(remoteUri);
var fileContent = await response.Content.ReadAsByteArrayAsync();
File.WriteAllBytes(fileName, fileContent);