In my case, you might want to use WeasyPrint (Vanilla). I use the Vanilla / Original Version because it's straightforward and easy to use.
Excerpt from my code in the repository that uses it. But I will also some demonstration anyway.
- Create an HTML View from where you want to render the content.
# inside pdfRenderer.html
{% load static %}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<meta http-equiv="x-ua-compatible" content="ie=edge">
<title>Simple Task Management | Summer Skilling #1</title>
</head>
<body>
{% include "databoard.html" with pdfRenderMode=True %}
</body>
<!-- ! Content Ends Here -->
</html>
Note: databoard.html
contains the layouts that I wanna render inside my PDF. Look at here instead. While pdfRender.html is the body where I wanna load databoard.html
inside.
- Use it like Normal Django Views but instead, we use WeasyPrint's functionalities to render it as a file.
# Inside views.py
from weasyprint import CSS, HTML
from django.template.loader import render_to_string
from django.core.files.storage import FileSystemStorage
from django.http import HttpResponse, HttpResponseRedirect
template_pdfRenderer = "pdfRenderer.html" # Refer to the html file I want to render at my PDF at.
# Create a Filename of the PDF.
pdfFileName = "%s_%s.pdf" % (
self.request.user.username,
datetime.datetime.now().strftime("%H%M%S%m%d%Y"),
)
# Renders The HTML with Model's Content.
ml_string = render_to_string(
template_pdfRenderer,
{"DataSets": UserTasks.objects.filter(Task_Owner=self.request.user.uuid)},
)
# Let WeasyPrint Get HTML String of ml_string.
# Input the location from where it would be saved (in server's part / perspective)
# Add some stylesheets incorporated with the HTMLs. DO NOT INCLUDE CSS INSIDE THESE TEMPLATES AS THEY WON'T WORK.
html = HTML(string=html_string).write_pdf(
target="userdata/ExportedPDFs/%s" % (pdfFileName),
stylesheets=[
CSS("CrudSimulation\static\css\material.min.css"),
CSS("CrudSimulation\static\css\sc_require-min.css"),
],
)
# Instantiate FileStorage.
fs = FileSystemStorage("")
# Open The Saved PDF and Let User Saved It By Packaging it to the HttpResponse.
with fs.open("userdata/ExportedPDFs/" + pdfFileName) as pdf:
response = HttpResponse(pdf, content_type="application/pdf")
response["Content-Disposition"] = 'attachment; filename="%s.pdf"' % (pdfFileName)
return response
# Once we're done, returning to a particular URL.
return HttpResponseRedirect(reverse("data_user_view"))
Result
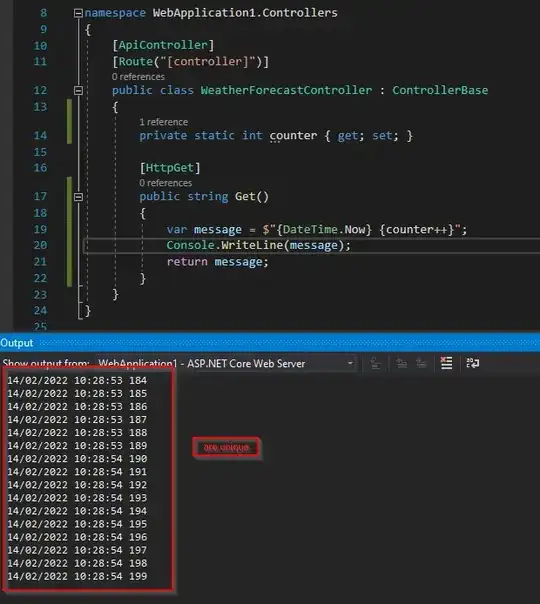
Output of the File.
Note: The rendering feels like it was saved via Print as PDF
but the flexibility of adding content is a nice addition as to why I recommended this.
For a basic setup, you might want to look at this guide > Using Python WeasyPrint generate HTML to PDF in django but, I primarily used this guide.