Impossible without unsafe in C # because you need pointers, you can create a function in C or C++ that meets your need and call it in C#.
With unsafe indeed you can handle a multidimensional arrays (Matrices) als 1D whith pointers - BUT you need to know at least the indentation and the count of all elements and it is valid only for arrays initialized like T[,,,], then you can take the example bellow:
public static void AddFiveOnMatrixArray(int[,,] numbers, int count)
{
unsafe
{
fixed (int* ptr = &numbers[0, 0, 0])
{
for (int i = 0; i < count; i++)
{
var x = *(ptr + i) + 5;
Console.Write($"{x} ");
}
Console.WriteLine();
}
}
}
but the above can be done for jagged arrays too whith some changes as follow:
public static void AddFiveOnJaggedArray(dynamic numbers)
{
if (numbers is Array && numbers[0] is Array)
{
for (int i = 0; i < numbers.Length; i++)
{
AddFiveOnJaggedArray(numbers[i]);
}
}
else
{
unsafe
{
fixed (int* ptr = &((int[])numbers)[0])
{
for (int i = 0; i < numbers.Length; i++)
{
var x = *(ptr + i) + 5;
Console.Write($"{x} ");
}
}
}
}
}
If you decide to use the second example, i dont know it may be make no sense to do your math operations whith pointers any more but it is up to you. If you have no info about the size of the array is probably better to iterate over it like above without pointers or anything and do your math over the elements instead of wrapping it into some other structure and then back again.
Hier is the Main method:
public static void Main()
{
Console.WriteLine("Math operations over array[,,]:");
AddFiveOnMatrixArray(new int[,,]
{
{
{ 10, 20, 30 },
{ 40, 50, 60 }
},
{
{ 70, 80, 90 },
{ 80, 70, 60 }
},
{
{ 50, 40, 30 },
{ 20, 10, 0 }
}
}, 18);
Console.WriteLine("Math operations over array[][][]:");
AddFiveOnJaggedArray(new int[][][]
{
new int[][]
{
new int[] { 10, 20, 30 },
new int[] { 40, 50, 60, 50, 40 }
},
new int[][]
{
new int[] { 70, 80, 90, 80, 70 },
new int[] { 80, 70, 60 }
},
new int[][]
{
new int[] { 50, 40, 30 },
new int[] { 20, 10, 0, 10, 20 }
}
});
}
and the output produced by both:
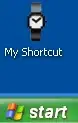