The link does answer it. All the answers clearly show that you should use push_back
(or insert
, actually), not put_child
.
You also have to read past the "how to make the array" and realize that you cannot have arrays as document root.
This is a symptom of the fact that Boost Ptree is not a JSON library. It's a Property Tree library, and it supports only property trees. The limitations are documented:
https://www.boost.org/doc/libs/1_74_0/doc/html/property_tree/parsers.html#property_tree.parsers.json_parser
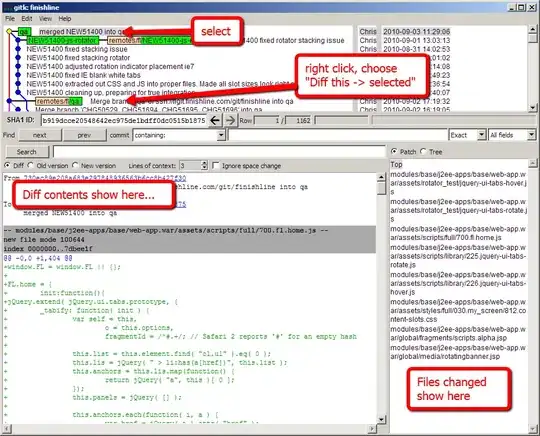
DEMO
Here's the best you can do, assuming you did not really need the array as document root:
Live On Coliru
#define BOOST_BIND_GLOBAL_PLACEHOLDERS
#include <boost/property_tree/json_parser.hpp>
#include <iostream>
using boost::property_tree::ptree;
int main() {
ptree arr;
for (auto [k,v]: { std::pair
{"3", "SomeValue"},
{"40", "AnotherValue"},
{"23", "SomethingElse"},
{"9", "AnotherOne"},
{"1", "LastOne"} })
{
ptree element;
element.put(k, v);
arr.push_back({"", element});
}
// can't have array at root of doc...
ptree doc;
doc.put_child("arr", arr);
write_json(std::cout, doc);
}
Prints
{
"arr": [
{
"3": "SomeValue"
},
{
"40": "AnotherValue"
},
{
"23": "SomethingElse"
},
{
"9": "AnotherOne"
},
{
"1": "LastOne"
}
]
}