I had a look at this tonight, as I expected it's throwing an InvalidOperationException
Relational-specific methods can only be used when the context is using a relational database provider.
These are common with the in-memory provider when you try to use relational methods like FromSql, ExecuteSqlCommand etc. Most of the time they can be mocked using a mock wrapper (I do exactly this for my library EntityFrameworkCore.Testing), it is often a lot of work though.
SetCommandTimeout is an extension. You'll need to dig into the extension and mock a few things on the database facade to get it to work.
However before we go down that path, mocking isn't going to work for you in this instance. When you mock an implementation the mocking library needs to be able to create an instance to proxy over, which means it executes the code in the constructor. SetCommandTimeout will execute on whatever the DbContextOptions you pass in generates during mock creation and once again barf.
On to your options:
Don't call set command timeout in your constructor; move it to another method, remember to invoke it etc
Use a different entity framework core provider that supports relational operations
Can't say I like the first option, but it would allow you to mock it. The second is far more palatable. I don't do a lot with SQLite but a quick play in LINQPad is yielding expected results:
void Main()
{
using (var connection = new SqliteConnection("Filename=:memory:"))
{
connection.Open();
var options = new DbContextOptionsBuilder<TestDbContext>().UseSqlite(connection).Options;
var testDbContext = new TestDbContext(options);
Console.WriteLine(testDbContext.Database.GetCommandTimeout());
}
}
public class TestDbContext : DbContext
{
public TestDbContext(DbContextOptions<TestDbContext> op) : base(op)
{
Database.SetCommandTimeout(9000);
}
}
Result:
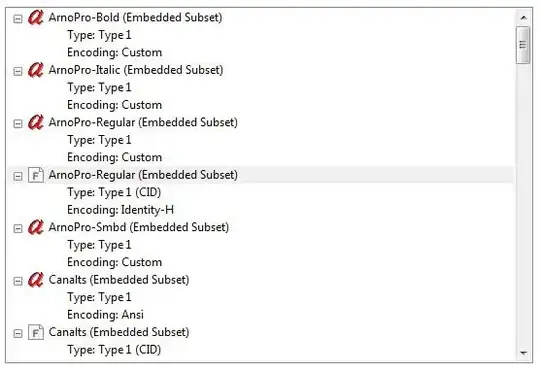