Unfortunatelly IntelliJ regex processor does not allow multiple backreferences per matched group and will capture only the last value for that particular group, therefore you could achieve your goal by processing each file part by part. I find this approach time consuming yet safe enough as you can verify replacements at place.
Here is a demo of how could one achieve the goal:
This will be the sample class that I'm using:
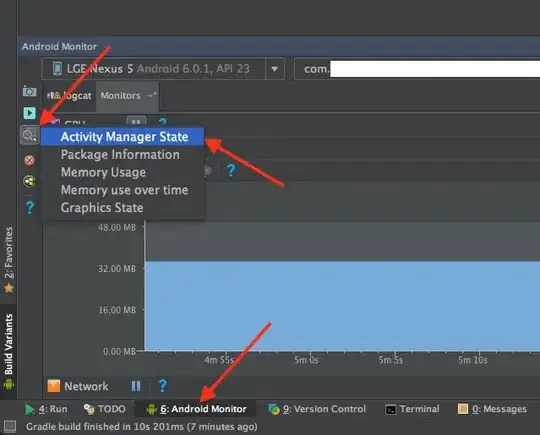
- Search & Replace all occurrances for regex pattern (project wise - enforces build failures and will highlight all the places that has to be fixed. A good idea is to check all before continuing forward):
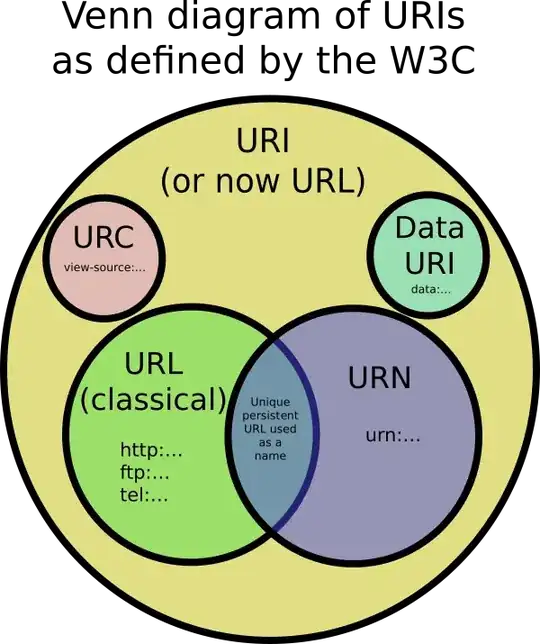
Steps from 2 and 3 should be repeated per declaration block individually!
Select the block that contains the put declarations and Search & Replace for regex pattern(selection wise - the screenshot that I added does this class wise but it isn't correct!)
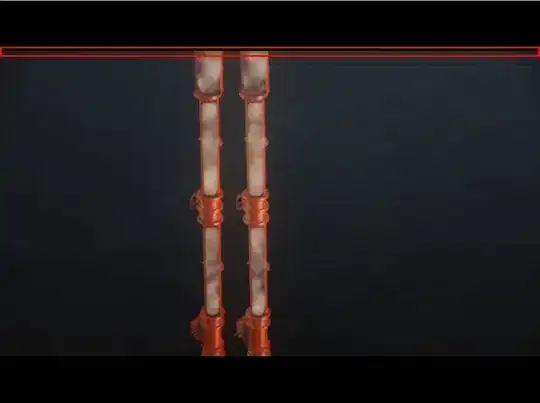
Select the closing curly brackets and manually fix them.
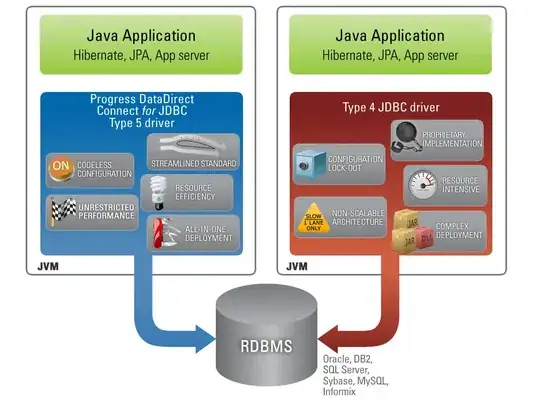
Here is the code used for the demo and the resulting code:
import java.math.BigInteger;
import java.util.HashMap;
import java.util.Map;
public class Main
{
public static void main(String[] args)
{
Map<Object, Object> aMap = new HashMap<Object, Object>() {{
put("1", 'a');
put(2, "b");
put(BigInteger.valueOf(3L), new char[3]);
}};
}
}
transformed to:
import java.math.BigInteger;
import java.util.HashMap;
import java.util.Map;
public class Main
{
public static void main(String[] args)
{
Map<Object, Object> aMap = ImmutableMap.of(
"1", 'a', 2, "b", BigInteger.valueOf(3L), new char[3]);
}
}
Regex used for step 1: new HashMap<\w+,\s*\w+>\(\)\s*\{\{
-> ImmutableMap.of(
Regex used for step 2: put\((.*)\,\s*(.*)\)\;\s+
-> $1, $2,
You will have to manually fix class imports as well.
P.S. One could write a script to do this, but generally it's not a good practice to process your codebase programatically.