Always make sure that you don't violate the Terms of Use of the actually scraped service. Maybe you could achieve the desired result if you'd use their API? (https://rapidapi.com/Privatix/api/temp-mail)
If you are sure that you want to use browser automation and proceed with retrieveing the one-time password with puppeteer then: you can use page.$eval
method to retrieve text content of any element with a valid selector.
Note: what you've already copied from devtools as the selector is actually a selector, it is not mandatory to include a CSS class or element id. It is totally fine (even if it is a bit redundant).
E.g.:
const selector = 'body > main > div.container > div > div.col-sm-12.col-md-12.col-lg-12.col-xl-8 > div.tm-content > div > div.inboxWarpMain > div > div.inbox-data-content > div.inbox-data-content-intro > div:nth-child(13) > div > table > tbody > tr > td > div:nth-child(2) > table > tbody > tr > td > div > table > tbody > tr > td > table > tbody > tr:nth-child(3) > td > div'
const text = await page.$eval(selector, el => el.innerText)
console.log(text)
Output:
233-552
Edit
In case there are more than one elements the selector would match, you can use document.querySelectorAll
approaches like $$eval
or $$
then select the element on the first index [0]
.
In this exact use case the $
is occupied by jQuery, so it conflicts with chrome api's $
shorthand for querySelector
, see here:
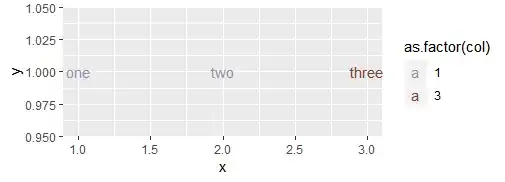
Solutions:
const selector = 'body > main > div.container > div > div.col-sm-12.col-md-12.col-lg-12.col-xl-8 > div.tm-content > div > div.inboxWarpMain > div > div.inbox-data-content > div.inbox-data-content-intro > div:nth-child(13) > div > table > tbody > tr > td > div:nth-child(2) > table > tbody > tr > td > div > table > tbody > tr > td > table > tbody > tr:nth-child(3) > td > div'
await page.waitFor(10000) // waitForTimeout since pptr 5.3.0
try {
await page.waitForSelector(selector)
const [text] = await page.$$eval(selector, elements => elements.map(el => el.innerText))
console.log(text)
} catch (e) {
console.error(e)
}
// alternate solution with page.evaluate:
try {
const text = await page.evaluate(el => el.innerText, (await page.$$(selector))[0])
console.log(text)
} catch (e) {
console.error(e)
}