You are are learning C# might as well jump right in and start developing classes that handle the logic required.
The trick is to start from the highest denomination note and count how many of them fit in the amount. For example
amount = 379
count_100 = amount/100 = 3 // integer division
amount = amount - count_100*100 // remainder 79
The move to the next largest note
count_50 = amount/50 = 1
amount = amount - count_50*50 // remainder 29
and proceed down to 20,10,5, and 1 notes.
The remainder is the change given out.
For example an BankNotes
class that splits up an amount to an array of note count for each denomination.
public struct BankNotes
{
public readonly static Decimal[] Notes = { 1m, 5m, 10m, 20m, 50m, 100m };
public static implicit operator BankNotes(decimal value) => new BankNotes(value);
public static implicit operator decimal(BankNotes amount) => amount.Value;
public BankNotes(decimal value)
{
// Initilize note counts to 0
this.NoteCount = new int[Notes.Length];
// Go from lagest note down to smallest
for (int i = NoteCount.Length - 1; i >= 0; i--)
{
// calc count for each note
NoteCount[i] = (int)(value / Notes[i]);
// adjust balance based on notes counted
value -= NoteCount[i] * Notes[i];
}
// set the remaining moneys
Remainder = value;
}
public decimal Remainder { get; }
// Array of count for each note.
public int[] NoteCount { get; }
// Total value of notes.
public decimal NotesValue
=> Notes.Zip(NoteCount, (note, count) => count * note).Sum();
// Tota value including remainder (for checK)
public decimal Value
=> NotesValue + Remainder;
}
And then to be used in a console application by handling the math for the user inputs
class Program
{
static void Main(string[] args)
{
bool done = false;
const decimal rate = 1.123m;
do
{
Console.WriteLine("Enter Amount in GBP");
string input = Console.ReadLine();
if (decimal.TryParse(input, out decimal amount))
{
amount = Math.Round(amount * rate, 2); // convert to EUR and round
Console.WriteLine($"Amount in EUR is {amount}");
BankNotes bank = new BankNotes(amount);
for (int i = 0; i < BankNotes.Notes.Length; i++)
{
if (bank.NoteCount[i] > 0)
{
Console.WriteLine($" {bank.NoteCount[i]} notes of {BankNotes.Notes[i]} EUR");
}
}
Console.WriteLine($" Sum : {bank.NotesValue} EUR");
Console.WriteLine($" Change: {bank.Remainder} EUR");
Console.WriteLine($" Error : {bank.Value - amount} EUR");
}
else
{
done = true;
}
} while (!done);
}
}
Here is a sample output, formatted a but differently though:
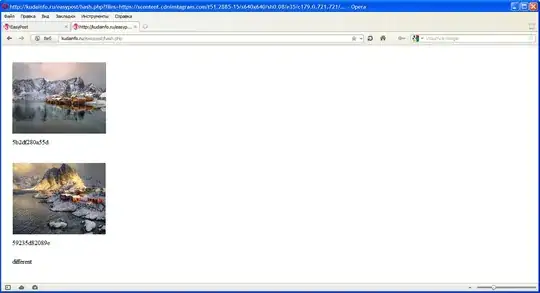