I'd use a UIStackView
inside each cell.
self.data = @[@[@"3", @"5", @"1"], @[@"12", @"6", @"7"], @[@"2", @"30", @"12"]];
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath {
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier: @"test" forIndexPath:indexPath];
UILabel *label1 = [UILabel new];
label1.text = self.data[indexPath.row][0];
label1.textAlignment = NSTextAlignmentCenter;
UILabel *label2 = [UILabel new];
label2.text = self.data[indexPath.row][1];
label2.textAlignment = NSTextAlignmentCenter;
UILabel *label3 = [UILabel new];
label3.text = self.data[indexPath.row][2];
label3.textAlignment = NSTextAlignmentCenter;
UIStackView *stackView = [[UIStackView alloc] initWithArrangedSubviews: @[label1, label2, label3]];
stackView.tag = 1;
stackView.axis = UILayoutConstraintAxisHorizontal;
stackView.distribution = UIStackViewDistributionFillEqually;
UIView *sub = [cell.contentView viewWithTag: 1];
if (sub != nil ) {
[sub removeFromSuperview];
}
[cell.contentView addSubview: stackView];
stackView.translatesAutoresizingMaskIntoConstraints = NO;
[stackView.centerYAnchor constraintEqualToAnchor: cell.contentView.centerYAnchor].active = YES;
[stackView.leadingAnchor constraintEqualToAnchor: cell.contentView.leadingAnchor constant: 10].active = YES;
[stackView.trailingAnchor constraintEqualToAnchor: cell.contentView.trailingAnchor constant: -10].active = YES;
return cell;
}
EDIT: Added a tag to the stack view to prevent cells that are reused to have more than one stack view.
result:
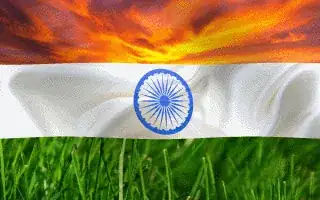