You can use $.fn.dataTable.ext.search.push()
- but it requires some additional work, to handle the fact (as you point out) that this is a global function, affecting all tables.
The following is not a full implementation, but shows the main points:
The Approach
My example uses 2 tables, with hard-coded data in each HTML table.
Each table is initialized as follows:
$(document).ready(function() {
// ---- first table with custom search ---------//
var table_a = $('#example_a').DataTable( {
pageLength: 10
} );
// remove the default DT search events - otherwise
// they will always fire before our custom event:
$( "#example_a_filter input" ).unbind();
// add our custom filter event for table_a:
$('#example_a_filter input').keyup( function( e ) {
table_a.draw();
} );
// ---- second table with DT default search ----//
var table_b = $('#example_b').DataTable( {
pageLength: 10
} );
} );
For my custom search function, I make use of the fact that the function includes a settings
parameter which we can use to see which table is being searched:
$.fn.dataTable.ext.search.push (
function( settings, searchData, index, rowData, counter ) {
var tableID = settings.nTable.id;
var searchTerm = $('#' + tableID + '_filter input').val()
//console.log( tableID );
//console.log( searchTerm );
//console.log( searchData );
switch(tableID) {
case 'example_a':
if (searchTerm === '') {
return true;
} else {
show_me = true;
searchData.forEach(function (item, index) {
if (show_me && item.includes(searchTerm)) {
show_me = false;
}
});
return show_me;
}
break;
default:
// for all other tables, pass through the rows
// already filtered by the default DT filter:
return true;
}
}
);
The following line is where we ID which table is being filtered:
var tableID = settings.nTable.id;
After that, I can use a switch
statement to handle each table's search separately.
In the default case (for example_b
), I'm just passing through what was already filtered by the DT default search:
default: return true;
The above filter looks like this when I search each table for the letter x
:
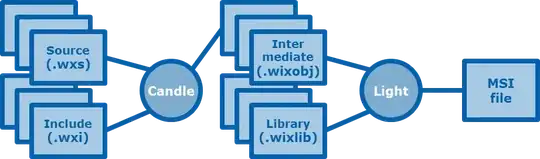
Incomplete Implementation
This logic is incomplete for the custom search logic. It assumes the search term is a single string. If I enter "x y" in the input field, that will exclude all records where a field contains "x y" - which is probably not what you want.
You probably want the exact negation of the standard search - so the input term would need to be split on spaces and each sub-term (x
and y
) would need to be checked separately across each row of data.