As already mentioned above, extend the control to have it drawn either horizontally or vertically.
Imports System.Drawing
Imports System.Drawing.Drawing2D
Imports System.Windows.Forms
Public Class ToggleSwitch
Inherits CheckBox
Public Sub New()
MyBase.New
SetStyle(ControlStyles.AllPaintingInWmPaint Or
ControlStyles.UserPaint, True)
UpdateStyles()
Padding = New Padding(6)
End Sub
Private _orientation As Orientation = Orientation.Horizontal
Public Property Orientation As Orientation
Get
Return _orientation
End Get
Set(value As Orientation)
_orientation = value
Dim max = Math.Max(Width, Height)
Dim min = Math.Min(Width, Height)
If value = Orientation.Vertical Then
Size = New Size(min, max)
Else
Size = New Size(max, min)
End If
End Set
End Property
'Fix by: @41686d6564
<Browsable(False),
Bindable(False),
DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden),
EditorBrowsable(EditorBrowsableState.Never)>
Public Overrides Property AutoSize As Boolean
Get
Return False
End Get
Set(value As Boolean)
End Set
End Property
Protected Overrides Sub OnPaint(e As PaintEventArgs)
Dim g = e.Graphics
g.Clear(BackColor)
g.SmoothingMode = SmoothingMode.AntiAlias
g.PixelOffsetMode = PixelOffsetMode.Half
Dim p = Padding.All
Dim r = 0
Dim rec As Rectangle
Using gp = New GraphicsPath
If _orientation = Orientation.Vertical Then
r = Width - 2 * p
gp.AddArc(p, p, r, r, -180, 180)
gp.AddArc(p, Height - r - p, r, r, 0, 180)
r = Width - 1
rec = New Rectangle(0, If(Checked, Height - r - 1, 0), r, r)
'Or
'rec = New Rectangle(0, If(Checked, 0, Height - r - 1), r, r)
'To get the ON on top.
Else
r = Height - 2 * p
gp.AddArc(p, p, r, r, 90, 180)
gp.AddArc(Width - r - p, p, r, r, -90, 180)
r = Height - 1
rec = New Rectangle(If(Checked, Width - r - 1, 0), 0, r, r)
End If
gp.CloseFigure()
g.FillPath(If(Checked, Brushes.DarkGray, Brushes.LightGray), gp)
g.FillEllipse(If(Checked, Brushes.Green, Brushes.WhiteSmoke), rec)
End Using
End Sub
End Class
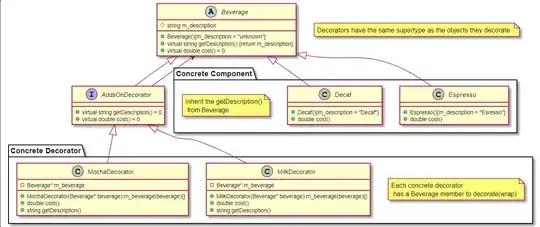
As for the second part of your question, please read CheckBox.Checked property and CheckBox.CheckedChanged event.
Impelemntation example:
Private Sub ToggleSwitch1_CheckedChanged(sender As Object, e As EventArgs) Handles ToggleSwitch1.CheckedChanged
If ToggleSwitch1.Checked Then
'ToDo with ON state...
Else
'ToDo with OFF state..
End If
End Sub