As mentioned in the comments, you can use ax.axhspan()
and ax.axvspan()
to add color to a specific background range. Arrows are set with ax.arrow(x,y,dx,dy)
, which draws an arrow alone. The sample solution is a customized version of the official reference. matplotlib.axes.Axes.arrow
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.dates as mdates
import matplotlib.cbook as cbook
years = mdates.YearLocator() # every year
months = mdates.MonthLocator() # every month
years_fmt = mdates.DateFormatter('%Y')
data = cbook.get_sample_data('goog.npz', np_load=True)['price_data']
fig, ax = plt.subplots(figsize=(12,9))
ax.plot('date', 'adj_close', data=data, color='g', lw=2.0)
ax.xaxis.set_major_locator(years)
ax.xaxis.set_major_formatter(years_fmt)
ax.xaxis.set_minor_locator(months)
datemin = np.datetime64(data['date'][0], 'Y')
datemax = np.datetime64(data['date'][-1], 'Y') + np.timedelta64(1, 'Y')
ax.set_xlim(datemin, datemax)
ax.format_xdata = mdates.DateFormatter('%Y-%m-%d')
ax.format_ydata = lambda x: '$%1.2f' % x # format the price.
# update
ax.axvspan(13514,13879, color='skyblue', alpha=0.2)
ax.arrow(13650,550,55,55, zorder=10, width=10, fc='b', ec='b')
ax.arrow(13930,630,25,-55, zorder=10, width=10, fc='red',ec='red')
fig.autofmt_xdate()
plt.show()
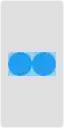