So, in order to scroll to multiple elements, you would need to use the driver.execute_script()
method. If you have your driver and you have your page opened, then you need to isolate the element that you want to navigate to.
element = driver.find_element(By.XPATH, "Whatever xpath you want")
driver.execute_script("return arguments[0].scrollIntoView();", element)
Once you have this, you will need to get a count of all elements that you want to scroll through. For example, in the Top 100 Greatest Movies List website, I created a method to get the current count of displayed movies
def get_movie_count(self):
return self.driver.find_elements(By.XPATH, "//div[@class='lister-list']//div[contains(@class, 'mode-detail')]").__len__()
After that, I created another method for scrolling to my element
def scroll_to_element(self, xpath : str):
element = self.driver.find_element(By.XPATH, xpath)
self.driver.execute_script("return arguments[0].scrollIntoView();", element)
Once that was done, I created a for-loop
and scrolled to each element
for row in range(movieCount):
driver.scroll_to_element("//div[@class='lister-list']//div[contains(@class, 'mode-detail')][{0}]".format(row + 1))
UPDATE: Added photo to help
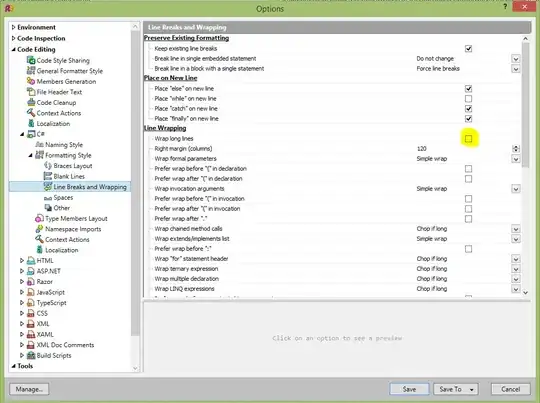
Photo Explanation
In this photo, we have our WebDriver
class. This is the class that creates our Chrome WebDriver and includes any options that we want. In my case, I did not want to see the "This is being controlled by automation" alert all the time.
In the WebDriver
class, I have a method called wait_displayed
that has selenium
check the browser's DOM
for a specific element using xpath
. If the element exists in the browser's DOM
, all is good. Otherwise, python
will raise an Exception
and let me know that selenium
could not find my element. In this class, I included the scroll_to_element
method which allows my chrome driver to find an element on the page and executes JavaScript
code to scroll to the element that was found.
In the SampleSite
class, we inherit the WebDriver
class. This means that my SampleSite
class can use the methods that display in my WebDriver
class. ( like wait_displayed
and scroll_to_element
) In the SampleSite
class, I provide an optional parameter
in it's constructor
method. ( __init__()
) This optional parameter tells the programmer that, if they want to create an instance
or object
of the SampleSite
class, they need to, either, pass in an existing chrome driver; or, pass in None
and the SampleSite
class will open Google Chrome and navigate to our Top 100 Greatest Movies Page. This is the class where I created the def get_movie_count(self):
method.
In our main_program.py
file, I imported our SampleSite
class. After import, I created an instance of our class and called the get_movie_count
method. Once that method returned my movie count ( 100 ), I created a for-loop
that scrolls through each of my elements.