Here is one approach to plot a sphere parametric surface as a wireframe:
import matplotlib.pyplot as plt
import mpl_toolkits.mplot3d as Axes3D
import numpy as np
def sphere():
# Parameters
R = 1
tht = np.linspace(0, 2* np.pi, 10)
phi = np.linspace(0, 2* np.pi, 10)
# Meshgrid to create mesh
tht, phi = np.meshgrid(tht, phi)
# Parametric equations in cartesian co-ordinates
x = (R * np.cos(tht))* np.cos(phi)
y = (R * np.cos(tht))* np.sin(phi)
z = R * np.sin(tht)
limx, limy, limz = (-2*R, 2*R), (-2*R, 2*R), (-2*R, 2*R)
return x, y, z, limx, limy, limz
# Adjustment for figure
fig = plt.figure('Parametric Surfaces')
ax = fig.add_subplot(111, projection='3d')
x, y, z, limx, limy, limz = sphere()
# Plot the data
h = ax.plot_wireframe(x, y, z, edgecolor='b')
# Add labels & title
ax.set_xlabel('X', fontweight = 'bold', fontsize = 14)
ax.set_ylabel('Y', fontweight = 'bold', fontsize = 14)
ax.set_zlabel('Z', fontweight = 'bold', fontsize = 14)
ax.set_title('Parametric Surface', fontweight = 'bold', fontsize = 16)
# Adjust figure axes
ax.set_xlim(*limx)
ax.set_ylim(*limy)
ax.set_zlim(*limz)
plt.show()
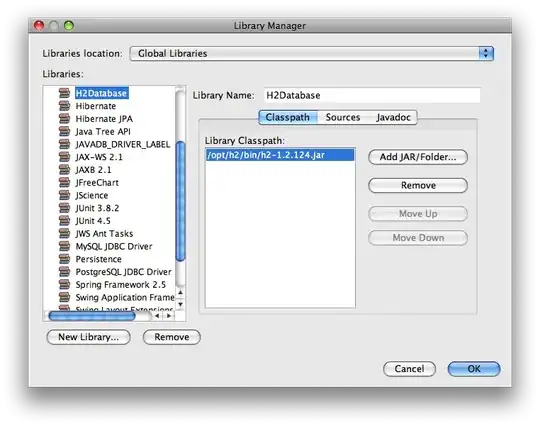