Lets assume your Box class is like this.
class Box
{
public int Id { get; set; }
public string Name { get; set; }
public string SrNo { get; set; }
public override string ToString()
{
return $"Id: {Id}\tName: {Name}\t SrNo: {SrNo}";
}
}
If you want to use linq, you can do it like this:
Box[] boxesOrganized =
boxes
.GroupBy(grp => grp.Name) //Create Grouping By Name
.ToArray()
.Select(s => new Box() //Create New Box For Each Grouping
{
Id = s.First().Id, //You Only Want The First Id
Name = s.First().Name, //You Only Want the First Name
SrNo = string.Join( //Grab The SrNo's And Seperate With A Comma
separator: ", ",
value: s.Select(s2 => s2.SrNo).ToArray())
})
.ToArray();
This will get you the output you were looking for:
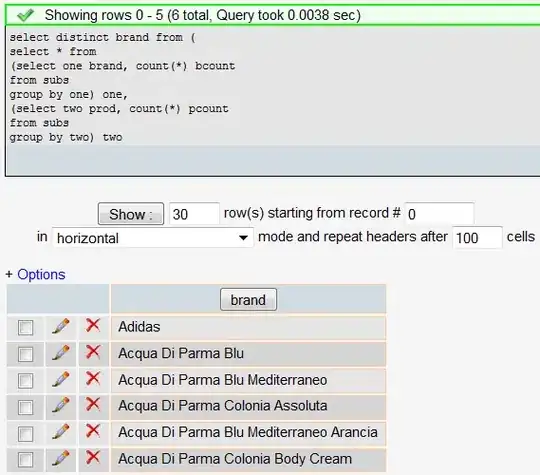
Full Code:
public static void GroupMyBoxes()
{
List<Box> boxes =
new List<Box>(){
new Box(){ Id=1, Name="A1", SrNo="A11"},
new Box(){ Id=2, Name="A1", SrNo="A22"},
new Box(){ Id=3, Name="B1", SrNo="B11"},
new Box(){ Id=4, Name="B1", SrNo="B22"},
new Box(){ Id=5, Name="C1", SrNo="C11"},
new Box(){ Id=6, Name="D1", SrNo="D11"}
};
Box[] boxesOrganized =
boxes
.GroupBy(grp => grp.Name) //Create Grouping By Name
.ToArray()
.Select(s => new Box() //Create New Box For Each Grouping
{
Id = s.First().Id, //You Only Want The First Id
Name = s.First().Name, //You Only Want the First Name
SrNo = string.Join( //Grab The SrNo's And Seperate With A Comma
separator: ", ",
value: s.Select(s2 => s2.SrNo).ToArray())
})
.ToArray();
//Print It Out To Verify
for (int i = 0; i < boxesOrganized.Length; i++)
{
Debug.WriteLine(""
+ $"{boxesOrganized[i].ToString()}");
}
}
In my experience I try to avoid Linq, as it can get somewhat difficult to follow. So I'll leave you with an alternative to keep it simple and have the same result.
public static void GroupWithDictionary() {
List<Box> boxes =
new List<Box>(){
new Box(){ Id=1, Name="A1", SrNo="A11"},
new Box(){ Id=2, Name="A1", SrNo="A22"},
new Box(){ Id=3, Name="B1", SrNo="B11"},
new Box(){ Id=4, Name="B1", SrNo="B22"},
new Box(){ Id=5, Name="C1", SrNo="C11"},
new Box(){ Id=6, Name="D1", SrNo="D11"}
};
Dictionary<string, List<Box>> boxDictionary =
new Dictionary<string, List<Box>>();
Box[][] _boxes =
new Box[][] { };
foreach (Box _box in boxes)
{
if (!boxDictionary.ContainsKey(_box.Name)) //If your box name doesn't exit
{ //Add It To The Dictionary
boxDictionary.Add(
key: _box.Name,
value: new List<Box>()); //Initilize A New List For the Box Name
}
boxDictionary[_box.Name].Add( //Now Add The Box To The List Of Boxes
item: _box); //With The Given Name
}
_boxes = //Dump Into An Array Of Box Arrays
boxDictionary //Or Whatever Suits Your Needs
.Select(s => s.Value.ToArray())
.ToArray();
//Print It Out To Verify
for (int i = 0; i < _boxes.Length; i++)
{
Debug.WriteLine(""
+ $"Id: {_boxes[i][0].Name}\t"
+$"Name: {_boxes[i][0].Name}\t"
+$"{string.Join(", ",_boxes[i].Select(s => s.SrNo))}");
}
}