If you do not have the original watermark image, then here is one way to mitigate the watermarks in Python/OpenCV using division normalization (divide a blurred copy of the image by the original image). It also whitens the background.
Input 1:
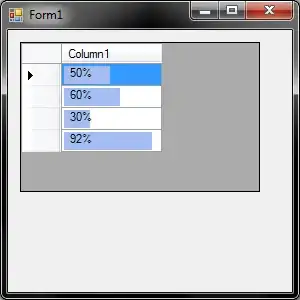
Input 2:
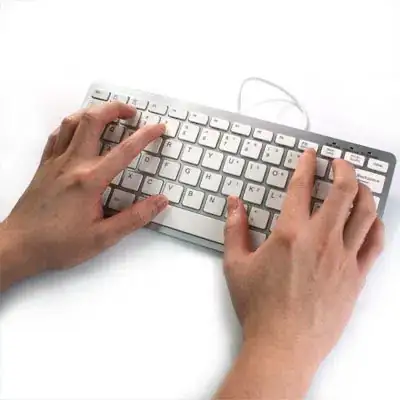
import cv2
import numpy as np
import skimage.exposure as exposure
import skimage.filters as filters
# read the image
img1 = cv2.imread('watermark1.jpg')
img2 = cv2.imread('watermark2.jpg')
# convert to gray
gray1 = cv2.cvtColor(img1,cv2.COLOR_BGR2GRAY)
gray2 = cv2.cvtColor(img2,cv2.COLOR_BGR2GRAY)
# blur
smooth1 = cv2.GaussianBlur(gray1, (5,5), 0)
smooth2 = cv2.GaussianBlur(gray2, (5,5), 0)
# divide gray by morphology image
division1 = cv2.divide(gray1, smooth1, scale=255)
division2 = cv2.divide(gray2, smooth2, scale=255)
# sharpen using unsharp masking
sharp1 = filters.unsharp_mask(division1, radius=3, amount=7, multichannel=False, preserve_range=False)
sharp1 = (255*sharp1).clip(0,255).astype(np.uint8)
sharp2 = filters.unsharp_mask(division2, radius=3, amount=9, multichannel=False, preserve_range=False)
sharp2 = (255*sharp2).clip(0,255).astype(np.uint8)
# save results
cv2.imwrite('watermark1_division_sharp.jpg',sharp1)
cv2.imwrite('watermark2_division_sharp.jpg',sharp2)
# show results
cv2.imshow('sharp1', sharp1)
cv2.imshow('sharp2', sharp2)
cv2.waitKey(0)
cv2.destroyAllWindows()
Result 1:
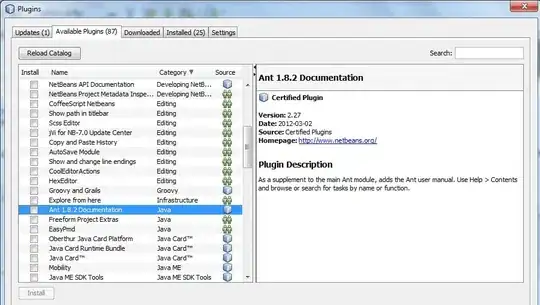
Result 2:
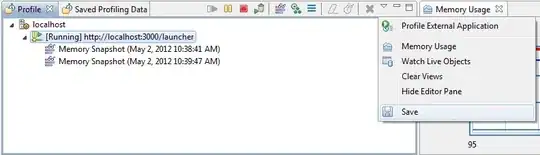