Whenever I rotate the device to landscape and back again, the top of the NavigationView
(including the title and back button) get clipped under the status bar.
Minimal reproducible example:
struct ContentView: View {
var body: some View {
NavigationView {
ScrollView {
VStack {
ForEach(0..<15) { _ in
Text("Filler")
.frame(maxWidth: .infinity)
.background(Color.green)
.padding()
}
}
}
.navigationBarTitle("Data")
}
}
}
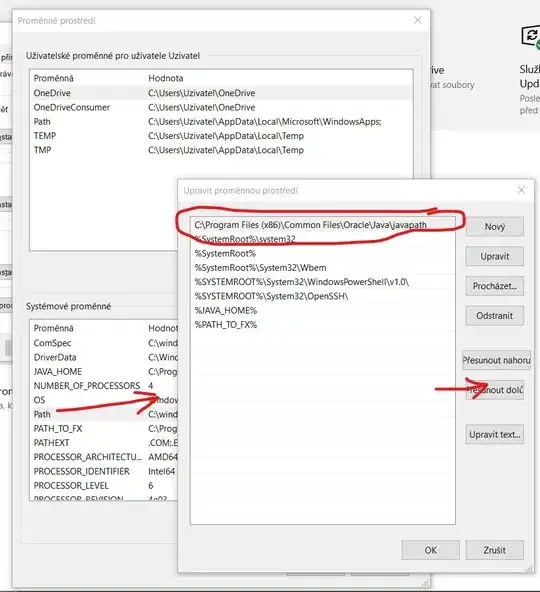
As long as the content is scrollable, the glitch happens. I originally came across this while using a List
. It didn't matter if I clicked a row first, went to the popped view, then rotated the device, or just rotated back in the main screen.
struct ContView: View {
let data = ["One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine", "Ten", "Eleven", "Twelve", "Thirteen", "Fourteen"]
var body: some View {
NavigationView {
List {
ForEach(data, id: \.self) { word in
NavigationLink(destination:
Text("Link")
) {
Text(word)
}
}
}
.navigationBarTitle("Data", displayMode: .large)
.listStyle(InsetGroupedListStyle())
}
}
}
Rotating in the main screen | Rotating after tapping a row |
---|---|
![]() |
![]() |
Is this a bug, or am I doing something wrong? I also get this printed in the console:
2020-10-19 09:05:30.613243-0700 MyAppName[43106:5613320] Unbalanced calls to begin/end appearance transitions for <TtGC7SwiftUI19UIHostingControllerGVS_15ModifiedContentVVS_22_VariadicView_Children7ElementGVS_18StyleContextWriterVS_19SidebarStyleContext__: 0x10340c180>.
Xcode version: 12.0 (12A7209), running on an iPhone 7 Plus on iOS 14.0