As @dwsolberg mentioned, Xcode has a new build setting called ENABLE_NS_ASSERTIONS
. For new projects its value for the release configuration is set to NO
and for all other configurations to YES
. You can use this setting as well as the widely used NS_BLOCK_ASSERTIONS
approach which is still valid in Xcode 6.
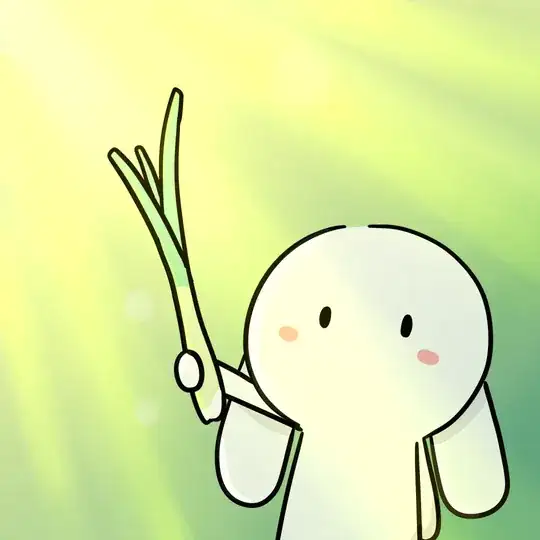
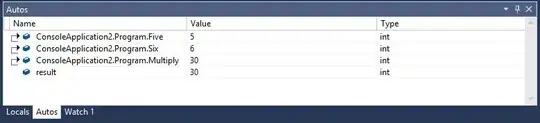
Assertions are a tool to track down bugs during development time and should never fire in productive code! Also exceptions should be used only if it is absoloutely neccessary, i.e. if something went so damn wrong that the programm is not able to continue execution. The Cocoa way is to give critical methods a boolean return value and parametrize them with an error object that can be set inside the method and can be used outside if the return value is NO
.
Hope that helps some folks ;-)