yes! you just plot your rose at lots of random points as sampled from your linked out question.
first I've refactored the method so it returns size
points uniformly sampled within the given triangle:
import numpy as np
import matplotlib.pyplot as plt
def trisample(A, B, C, size=1):
r1 = np.random.rand(size)
r2 = np.random.rand(size)
s1 = np.sqrt(r1)
p1 = 1 - s1
p2 = (1 - r2) * s1
p3 = r2 * s1
x = A[0] * p1 + B[0] * p2 + C[0] * p3
y = A[1] * p1 + B[1] * p2 + C[1] * p3
return x, y
next we calculate a few of your roses:
t, k, z = np.linspace(0, np.pi, 5*5+1), 5, 0.1
x_r = np.cos(k*t) * np.sin(t) * z
y_r = np.cos(k*t) * np.cos(t) * z
note that your pi*2
meant that it was orbited twice so I've dropped that, also I only use 5 points per "petal" to speed things up. z
scales the roses down so they fit into the triangle
finally we sample some points in the triangle, and plot them as you did:
for x_t, y_t in zip(*trisample([1,1], [5,3], [2,5], 100)):
plt.plot(x_r + x_t, y_r + y_t, lw=1)
plt.axis('off')
plt.axis('square');
which gives something like the following:
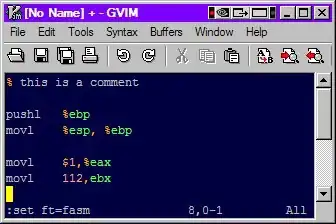