I have an .obj file loaded with THREE.OBJLoader in my three.js scene.
I'd like to apply a linear color gradient on the z-axis onto this object while preserving MeshStandardMaterial shaders. An example of a 2 color linear gradient applied onto an object is below.
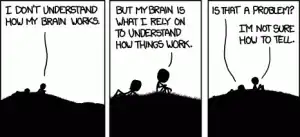
I need to do this while preserving the shaders that come with the MeshStandardMaterial class. I think to do this, I need to inject the vertexShader and materialShaders of MeshStandardMaterial with some code.
This is partially achieved in the answers to this question. These answers create a new shader material without the properties of the MeshStandardMaterial, which I neeed.
I tried using onBeforeCompile but I don't know where to inject the code that is outlined in the answers to the question I mentioned above. I only have a basic understanding of shaders.
The material of my object is defined by the THREE.MeshStandardMaterial class. I set the following properties of the class: metalness, roughness, transparent and opacity. I set the map and envmap properties with textures.