I would suggest you to learn how to debug your code when it is not working, this is still the best way to learn IMHO.
You could for example use an online assembler/debugger/emulator such as the cpulator.
copy/paste the code you want to test,
assemble it,
step into it while looking at the register values, memory content while your program is being executed step by step.
.global _start
_start:
ldr r0,= first
ldr r1,= last
ldr r2,= dst
// The CopyMemory function receives the following parameters:
// R0: Address of the first 32-bit word to copy
// R1: Address of the last 32-bit word to copy
// R2: Destination address
add r4, r1, #4
loop:
cmp r4, r1
beq endloop
ldr r3, [r0]
str r3, [r2]
add r0, r0, #4
add r2, r2, #4
b loop
endloop:
b .
.data
first: .word 0x11111111
.word 0x22222222
.word 0x33333333
last: .word 0x44444444
dst: .word 0x00000000
.word 0x00000000
.word 0x00000000
.word 0x00000000
.end
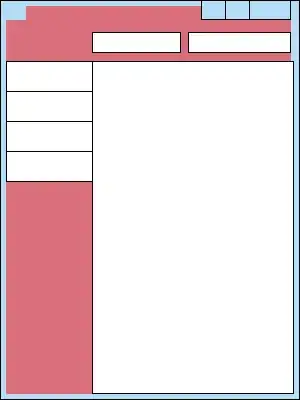
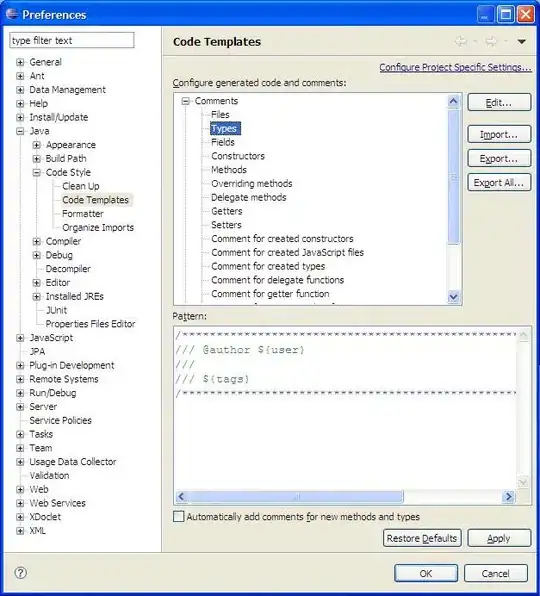
If you are a Windows user and don't know anything about Linux, an alternative would be to use Windows versions of qemu-system-arm
and GDB
, but this would probably be more difficult to do for you at this stage.
They could be downloaded respectively from here and here.
If you are familiar/using a Linux system, using qemu-user
as suggested by Peter Cordes in his comment would be a good solution as well. This could be done on a native Linux system, or by using a VirtualBox
virtual machine on Windows, or by using one of the WSL
or WSL2
Windows subsystems.