There are several ways to go about it, but it will also depend on whether you have:
- Multiple
<ul />
s
- Single
<ul />
with only 2 dropdowns
- Single
<ul />
with multiple dropdowns
Multiple <ul />
s
If you can change its layout, you can break up the dropdowns into 2 .navbar-nav
, and set justify-content
as space-between
on .navbar-collapse
so that the right .navbar-nav
will be pushed to the right, since .navbar-collapse
has already been displayed as flex-box:
<nav class="navbar navbar-expand-lg navbar-dark bg-dark">
<a class="navbar-brand" href="#">Navbar</a>
<button />
<div class="collapse navbar-collapse justify-content-lg-between">
<ul class="navbar-nav">
<li class="nav-item dropdown">
<a class="nav-link dropdown-toggle" />
<div class="dropdown-menu" />
</li>
</ul>
<ul class="navbar-nav">
<li class="nav-item dropdown">
<a class="nav-link dropdown-toggle" />
<div class="dropdown-menu dropdown-menu-right" />
</li>
</ul>
</div>
</nav>

demo: https://jsfiddle.net/davidliang2008/62sf4kvr/19/
Documentation for Bootstrap flex-box built-in classes: https://getbootstrap.com/docs/4.5/utilities/flex/
Single <ul />
with only 2 dropdowns
If you can't change the layout and have to go with a single <ul />
approach, and you only have 2 dropdowns, you can apply the same concept on .navbar-nav
instead of .navbar-collapse
: to set justify-content: between;
. The only additional thing you would need to do is to set the width of .navbar-nav
to 100%.
<nav class="navbar navbar-expand-lg navbar-dark bg-dark">
<a class="navbar-brand" href="#">Navbar</a>
<button />
<div class="collapse navbar-collapse">
<ul class="navbar-nav w-100 justify-content-lg-between">
<li class="nav-item dropdown">
<a class="nav-link dropdown-toggle" />
<div class="dropdown-menu" />
</li>
<li class="nav-item dropdown">
<a class="nav-link dropdown-toggle" />
<div class="dropdown-menu dropdown-menu-right" />
</li>
</ul>
</div>
</nav>
demo: https://jsfiddle.net/davidliang2008/62sf4kvr/23/
Single <ul />
with multiple dropdowns
If you have multiple dropdowns but only want to put the last one to the right, while the rest will align to the left, the above approaches won't work because they use justify-content: between;
for the effects.
In order to do that with multiple dropdowns, you can set the margin left of the last dropdown to auto, which will push itself to the furthest to the right:
<nav class="navbar navbar-expand-lg navbar-dark bg-dark">
<a class="navbar-brand" href="#">Navbar</a>
<button />
<div class="collapse navbar-collapse">
<ul class="navbar-nav w-100">
<li class="nav-item dropdown">
<a class="nav-link dropdown-toggle" />
<div class="dropdown-menu" />
</li>
<li class="nav-item dropdown">
<a class="nav-link dropdown-toggle" />
<div class="dropdown-menu" />
</li>
<li class="nav-item dropdown ml-lg-auto">
<a class="nav-link dropdown-toggle" />
<div class="dropdown-menu dropdown-menu-right" />
</li>
</ul>
</div>
</nav>
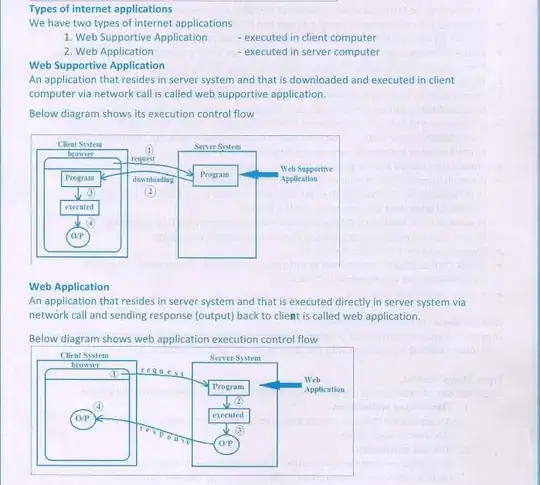
demo: https://jsfiddle.net/davidliang2008/62sf4kvr/26/