Inherited Graphics
image drawing methods
drawImage(Image img, int x, int y, Color bgcolor, ImageObserver observer)
drawImage(Image img, int x, int y, ImageObserver observer)
drawImage(Image img, int x, int y, int width, int height, Color bgcolor, ImageObserver observer)
drawImage(Image img, int x, int y, int width, int height, ImageObserver observer)
drawImage(Image img, int dx1, int dy1, int dx2, int dy2, int sx1, int sy1, int sx2, int sy2, Color bgcolor, ImageObserver observer)
drawImage(Image img, int dx1, int dy1, int dx2, int dy2, int sx1, int sy1, int sx2, int sy2, ImageObserver observer)
Choose your poison. Since you weren't even able to locate these, I'm assuming that going into detail about Intermediate Images when faced with scaling and frequent rendering would be futile.
Example 1 -- drawing a circle in a square
public class DrawCircleInSquare {
public static void main(String[] args){
SwingUtilities.invokeLater(new Runnable(){
@Override
public void run() {
createAndShowGUI();
}
});
}
private static void createAndShowGUI(){
final JFrame frame = new JFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
final JPanel panel = new JPanel(){
@Override
protected void paintComponent(Graphics g){
Graphics2D g2 = (Graphics2D)g.create();
// Clear background to white
g2.setColor(Color.WHITE);
g2.clearRect(0, 0, getWidth(), getHeight());
// Draw square
g2.setColor(Color.BLACK);
g2.drawRect(50, 50, 100, 100);
// Draw circle inside square
g2.setColor(Color.RED);
g2.fillOval(88, 88, 24, 24);
g2.dispose();
}
@Override
public Dimension getPreferredSize(){
return new Dimension(200, 200);
}
};
frame.add(panel);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
}
Output

Example 2 -- draw an image in a square
public class DrawImageInSquare {
private static BufferedImage bi;
public static void main(String[] args){
try {
// Load image
loadImage();
// Create and show GUI
SwingUtilities.invokeLater(new Runnable(){
@Override
public void run() {
createAndShowGUI();
}
});
} catch (IOException e) {
// handle exception
}
}
private static void loadImage() throws IOException{
bi = ImageIO.read(new File("src/resources/psyduck.png"));
}
private static void createAndShowGUI(){
final JFrame frame = new JFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
final JPanel panel = new JPanel(){
@Override
protected void paintComponent(Graphics g){
Graphics2D g2 = (Graphics2D)g.create();
// Clear background to white
g2.setColor(Color.WHITE);
g2.clearRect(0, 0, getWidth(), getHeight());
// Draw square
g2.setColor(Color.BLACK);
g2.drawRect(50, 50, 100, 100);
// Draw image inside square
g2.setRenderingHint(
RenderingHints.KEY_INTERPOLATION,
RenderingHints.VALUE_INTERPOLATION_BICUBIC);
g2.drawImage(bi, 50, 50, 100, 100, null);
g2.dispose();
}
@Override
public Dimension getPreferredSize(){
return new Dimension(200, 200);
}
};
frame.add(panel);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
}
Output
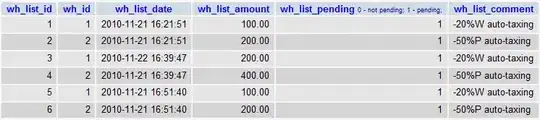