Based on the answer: https://stackoverflow.com/a/57023183/1730895
It's fairly easy to calculate the intersection of the rectangles:
def rectIntersection( rect1, rect2 ):
""" Given two pygame.Rect intersecting rectangles, return the overlap rect """
left = max( rect1.left, rect2.left )
width = min( rect1.right, rect2.right ) - left
top = max( rect1.top, rect2.top )
height= min( rect1.bottom, rect2.bottom ) - top
return pygame.Rect( left, top, width, height )
So use pygame.rect.colliderect()
(doco) first to determine if there's actually any overlap. Obviously this gives you an overlapping region, so to answer your question, it could be every pixel within this rectangular area. But perhaps you could just take the centroid for use as a "happening at" point.
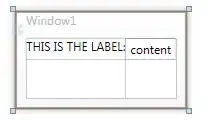
import pygame
# Window size
WINDOW_WIDTH = 400
WINDOW_HEIGHT = 400
WINDOW_SURFACE = pygame.HWSURFACE|pygame.DOUBLEBUF|pygame.RESIZABLE
WINDOW_MAXFPS = 60
# Colours
DARK_BLUE = ( 3, 5, 54)
RED = (255, 0, 0)
YELLOW = (200, 200, 20)
GREEN = ( 20, 200, 20)
def rectIntersection( rect1, rect2 ):
""" Given two pygame.Rect intersecting rectangles, calculate the rectangle of intersection """
left = max( rect1.left, rect2.left )
width = min( rect1.right, rect2.right ) - left
top = max( rect1.top, rect2.top )
height= min( rect1.bottom, rect2.bottom ) - top
return pygame.Rect( left, top, width, height )
### Initialisation
pygame.init()
pygame.mixer.init()
window = pygame.display.set_mode( ( WINDOW_WIDTH, WINDOW_HEIGHT ), WINDOW_SURFACE )
pygame.display.set_caption("Rect-Rect Intersection")
central_rect = pygame.Rect( 175, 175, 50, 50 )
player_rect = pygame.Rect( 0, 0, 30, 30 )
### Main Loop
clock = pygame.time.Clock()
done = False
while not done:
# Handle user-input
for event in pygame.event.get():
if ( event.type == pygame.QUIT ):
done = True
elif ( event.type == pygame.MOUSEMOTION ): # move the player's rectangle with the mouse
mouse_pos = pygame.mouse.get_pos()
player_rect.center = mouse_pos
# Update the window
window.fill( DARK_BLUE )
pygame.draw.rect( window, RED, central_rect, 1 ) # centre rect
pygame.draw.rect( window, YELLOW, player_rect, 1 ) # player's mouse rect
# IFF the rectangles overlap, paint the overlap
if ( player_rect.colliderect( central_rect ) ):
overlap_rect = rectIntersection( player_rect, central_rect )
pygame.draw.rect( window, GREEN, overlap_rect )
pygame.display.flip()
# Clamp FPS
clock.tick_busy_loop( WINDOW_MAXFPS )
pygame.quit()
If pixel-perfect collision is desired, use Surface.subsurface()
with the overlap rectangle's co-ordinates on the bitmap mask of both sprites. This gives you two bit-masks (maskA and maskB) which you know overlap somehow.
I can't think of a fast way to find the exact pixels of overlap between these two sub-sections. However it's quite easy to iterate through each of the mask-pixels, finding maskA & maskB pairs that are both "on". Obviously you need to convert from screen co-ordinates to sprite co-ordinates during this step, but this is just a subtraction of the sprite's current screen x
and y
.
What you really want is the "edge" of the bitmap-intersection, but that's another question.