New in iOS 14 is LazyVGrid which might help. Here is an example using your Interest pill.
struct WrappedPills: View {
let interests = [
"Photographs",
"Travel",
"Reading",
"Gardening",
"Cooking",
"Painting",
"Dancing",
"Music",
"Hiking",
"Art",
"Astronomy",
"Surfing",
"Knitting",
]
// let columns = [ GridItem(.adaptive(minimum: 100)) ]
let columns = [
GridItem(.flexible()),
GridItem(.flexible()),
GridItem(.flexible()),
]
var body: some View {
ScrollView() {
HStack {
Text("Interests")
.font(.largeTitle)
.fontWeight(.semibold)
.padding(.top)
.padding(.leading, 20)
Spacer()
}
LazyVGrid(
columns: columns,
alignment: .leading,
spacing: 10
){
ForEach(interests, id: \.self) { interest in
Text(interest)
.padding(.horizontal, 10)
.padding(.vertical, 5)
.background(Color.blue)
.foregroundColor(Color.white)
.cornerRadius(25.0)
.lineLimit(1)
.fixedSize(horizontal: true, vertical: true)
} //ForEach
} //LazyVGrid
} //Scrollview
.padding()
}
}
You can try a few other options with the "columns" arrangement that might suit your needs better.
Paul Hudson has some good examples of LazyVGrid here:
https://www.hackingwithswift.com/quick-start/swiftui/how-to-position-views-in-a-grid-using-lazyvgrid-and-lazyhgrid
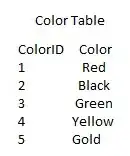