What I did in my application is just called player1.Stop();
when the Windows is going to suspend mode.
MainWindow.xaml
<Window ...
Loaded="Window_Loaded">
<Grid Margin="5">
<Grid.RowDefinitions>
<RowDefinition Height="*" />
<RowDefinition Height="*" />
<RowDefinition Height="*" />
</Grid.RowDefinitions>
<Button Click="Button_PlayResume" >Play/Resume</Button>
<Button Click="Button_Pause" Grid.Row="1">Pause</Button>
</Grid>
</Window>
MainWindow.xaml.cs
public partial class MainWindow : Window
{
MediaPlayer player1;
// Last play position before going to suspend mode
private TimeSpan LastPosition = TimeSpan.Zero;
public MainWindow()
{
InitializeComponent();
player1 = new MediaPlayer();
player1.MediaEnded += Player1_MediaEnded;
}
private void OpenMedia()
{
player1.Open(new Uri(@"M:\Sting.wav"));
//player1.Open(new Uri(@"M:\ring.wav"));
}
private void Player1_MediaEnded(object sender, EventArgs e)
{
LastPosition = TimeSpan.Zero;
}
private void Button_PlayResume(object sender, RoutedEventArgs e)
{
if (LastPosition != TimeSpan.Zero)
{
player1.Position = LastPosition; // Resume
LastPosition = TimeSpan.Zero;
}
else
{
OpenMedia();
}
player1.Play();
}
private void Button_Pause(object sender, RoutedEventArgs e)
{
player1.Pause();
LastPosition = player1.Position;
}
private void Window_Loaded(object sender, RoutedEventArgs e)
{
SystemEvents.PowerModeChanged += OnPowerModeChanged;
}
private void OnPowerModeChanged(object sender, PowerModeChangedEventArgs e)
{
if (e.Mode == PowerModes.Suspend)
{
if (player1.CanPause)
{
player1.Pause();
LastPosition = player1.Position;
}
player1.Stop();
}
}
}
The screen-shot:
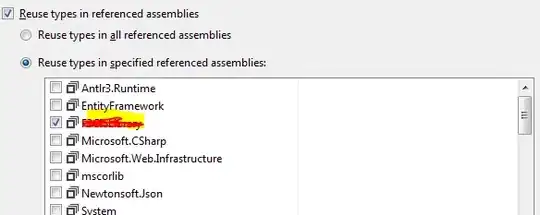
This will work even when the MediaPlayer
is playing something.
After resuming from sleeping mode press on Play/Resume button to continue playing from the latest position.