Edit:
I've seen the console log for the 'message' object that you have shared in the comment and below is my update from that:
- As we can see from the below image, message.member is 'null' due to which you were getting the error that you cannot find the property 'roles' of undefined (member which is null here).

- I believe what you are looking for here is
message.guild.roles
here which is a collection (Map). Please see the screenshot below.
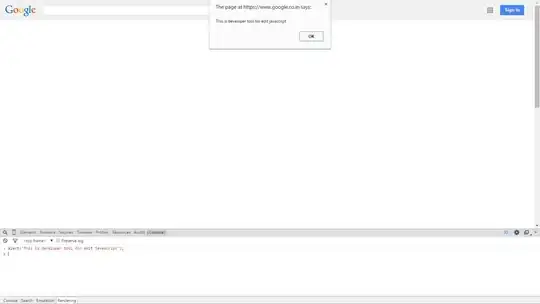
You should try to retrieve this roles Map and iterate over it for the in the following way:
const roles = message.guild.roles;
You can then use roles.entries()
in order to get the key-value pairs from the roles MAP and iterate over them and use the if condition that you were initially using.
Like the error message states, this is happening because 'member' is undefined. You can either have a guard clause like mentioned by user Paulquappe in his answer, but the guard clause would be for member and not roles, so something like this:
if (message.member && message.member.roles.some(role => role.name === 'role'))
or, if you are going to transpile your code, you can use optional chaining from ES2020 (this is a very new feature in JS, therefore I would not recommend it unless you are going to transpile your code):
The optional chaining operator acts like a guard clause, but the syntax only requires that you use a '?' before chaining using the dot syntax.
if (message.member?.roles.some(role => role.name === 'role'))