In case someone is stumbling over this: I have a similar usecase where I want to take advantage of jib (Dockerfile-less + can be used without docker daemon), but I currently cannot turn away from fat jars for other reasons.
Based on Chanseok comments (under the question), I came up with following, which will:
- drop any layers added by jib
- add the fatjar (by default in
target/${build.finalName}.jar
) into its own layer
- overwrite entrypoint to execute "java -jar ..." instead of regular entrypoint pointing to your Main
- Jib is called explicitly and not bound to lifecycle (at least in my sample), so it would have to be called explicitly with following
mvn package jib:dockerBuild
(or ... jib:build
for a docker-daemon-less build)
- using dive, you see that only one layer was added and that it is fairly large (== fat jar)
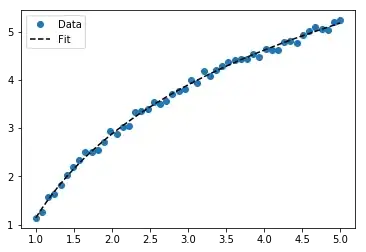
Sources: https://gitter.im/google/jib?at=5fad68c5d37a1a13d6a12174 + https://github.com/GoogleContainerTools/jib/tree/master/jib-maven-plugin#extradirectories-object
That sample works at least with spring boot 2.4.X
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
<plugin>
<groupId>com.google.cloud.tools</groupId>
<artifactId>jib-maven-plugin</artifactId>
<version>3.0.0</version>
<dependencies>
<dependency>
<groupId>com.google.cloud.tools</groupId>
<artifactId>jib-layer-filter-extension-maven</artifactId>
<version>0.2.0</version>
</dependency>
</dependencies>
<configuration>
<container>
<entrypoint>java,-jar,/app/${project.build.finalName}.jar</entrypoint>
</container>
<extraDirectories>
<paths>
<path>
<from>target/</from>
<includes>*.jar</includes>
<into>/app</into>
</path>
</paths>
</extraDirectories>
<pluginExtensions>
<pluginExtension>
<implementation>com.google.cloud.tools.jib.maven.extension.layerfilter.JibLayerFilterExtension</implementation>
<configuration implementation="com.google.cloud.tools.jib.maven.extension.layerfilter.Configuration">
<filters>
<filter>
<!-- exclude all jib layers, which is basically anything in /app -->
<glob>/app/**</glob>
</filter>
<filter>
<!-- this is our fat jar, this should be kept by adding it into its own layer -->
<glob>/app/${project.build.finalName}.jar</glob>
<toLayer>jib-custom-fatJar</toLayer>
</filter>
</filters>
</configuration>
</pluginExtension>
</pluginExtensions>
</configuration>
</plugin>
</plugins>
</build>