If I understand well, you can use a simply trick: add a containerView and do your stuff in it and assign the color that you want to dummy status bar simply changing the background color of the view:
declare containerView and the label in it:
let containerView = UIView()
let labelInfo = UILabel()
now in viewDidLoad set objects and constraints:
view.backgroundColor = .green // change color for status bar color
labelInfo.text = "do your stuff here"
labelInfo.font = .systemFont(ofSize: 24, weight: .semibold)
labelInfo.textColor = .black
labelInfo.textAlignment = .center
labelInfo.translatesAutoresizingMaskIntoConstraints = false
containerView.backgroundColor = .lightGray
containerView.translatesAutoresizingMaskIntoConstraints = false
view.addSubview(containerView)
containerView.topAnchor.constraint(equalTo: view.safeAreaLayoutGuide.topAnchor).isActive = true
containerView.bottomAnchor.constraint(equalTo: view.safeAreaLayoutGuide.bottomAnchor).isActive = true
containerView.leadingAnchor.constraint(equalTo: view.safeAreaLayoutGuide.leadingAnchor).isActive = true
containerView.trailingAnchor.constraint(equalTo: view.safeAreaLayoutGuide.trailingAnchor).isActive = true
containerView.addSubview(labelInfo)
labelInfo.trailingAnchor.constraint(equalTo: containerView.trailingAnchor).isActive = true
labelInfo.leadingAnchor.constraint(equalTo: containerView.leadingAnchor).isActive = true
labelInfo.heightAnchor.constraint(equalToConstant: 50).isActive = true
labelInfo.centerYAnchor.constraint(equalTo: containerView.centerYAnchor).isActive = true
this is the results:
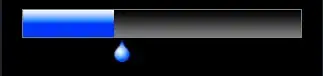