It produces error because delegation functions of MutableState
are extension functions because of that they need to be imported.
/**
* Permits property delegation of `val`s using `by` for [State].
*
* @sample androidx.compose.runtime.samples.DelegatedReadOnlyStateSample
*/
@Suppress("NOTHING_TO_INLINE")
inline operator fun <T> State<T>.getValue(thisObj: Any?, property: KProperty<*>): T = value
/**
* Permits property delegation of `var`s using `by` for [MutableState].
*
* @sample androidx.compose.runtime.samples.DelegatedStateSample
*/
@Suppress("NOTHING_TO_INLINE")
inline operator fun <T> MutableState<T>.setValue(thisObj: Any?, property: KProperty<*>, value: T) {
this.value = value
}
/**
* A mutable value holder where reads to the [value] property during the execution of a [Composable]
* function, the current [RecomposeScope] will be subscribed to changes of that value. When the
* [value] property is written to and changed, a recomposition of any subscribed [RecomposeScope]s
* will be scheduled. If [value] is written to with the same value, no recompositions will be
* scheduled.
*
* @see [State]
* @see [mutableStateOf]
*/
@Stable
interface MutableState<T> : State<T> {
override var value: T
operator fun component1(): T
operator fun component2(): (T) -> Unit
}
With
import androidx.compose.runtime.getValue
import androidx.compose.runtime.setValue
getValue
and setValue
functions are imported.
With Kotlin delegation you can either create delegation functions as part of the class
/**
* Delegate [MyState] to this function
*/
fun myStateOf() = MyState()
class MyState internal constructor() {
var value = 0
operator fun getValue(thisRef: Any?, property: KProperty<*>): Int = value
operator fun setValue(thisRef: Any?, property: KProperty<*>, value: Int) {
this.value = value
}
}
then use them without the need of importing since they are part of the class
var myState by myStateOf()
myState = 5
or define them as extension functions which is the case with State
and MutableState
interfaces.
/**
* Delegate [MyState] to this function
*/
fun myStateOf() = MyState()
class MyState internal constructor() {
var value = 0
}
@Suppress("NOTHING_TO_INLINE")
inline operator fun MyState.getValue(thisRef: Any?, property: KProperty<*>): Int = value
@Suppress("NOTHING_TO_INLINE")
inline operator fun MyState.setValue(thisObj: Any?, property: KProperty<*>, value: Int) {
this.value = value
}
When you try to call
var myState by myStateOf()
myState = 5
you get error ask you to import getValue and setValue functions.
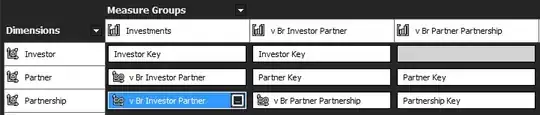