Try this approach. You can smartly arrange your data, group by time and create a y coordinate based on row number. With that you can sketch the plot. The average line and its label can be added with annotate()
and geom_vline()
. Here the code using geom_text()
and some tidyverse
functions:
library(dplyr)
library(ggplot2)
#Plot
df %>%
arrange(Time) %>%
group_by(Time) %>%
mutate(y=row_number()) %>%
ggplot(aes(x=Time))+
geom_text(aes(y=y,label=ID),size=3,fontface='bold')+
geom_vline(data=df%>% summarise(Time=mean(Time)),aes(xintercept=Time),color='red')+
theme_bw()+
annotate(geom='text',
x=df%>% summarise(Time=mean(Time)) %>% pull(Time)+3,
label=paste0('Avg is ',df%>% summarise(Time=mean(Time)) %>% pull(Time)),
y=7,fontface='bold',size=3)
Output:
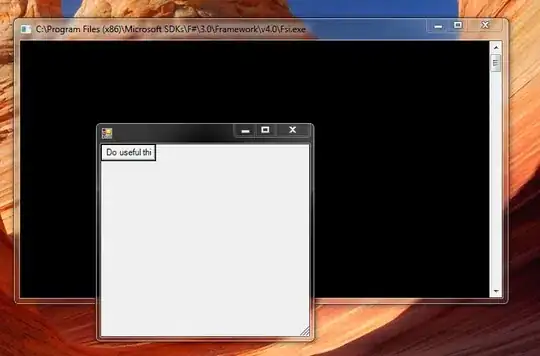
And if you want to go around perfection in terms of customization:
#Plot 2
df %>%
arrange(Time) %>%
group_by(Time) %>%
mutate(y=row_number()) %>%
ggplot(aes(x=Time))+
geom_text(aes(y=y,label=ID),size=3,fontface='bold')+
geom_vline(data=df%>% summarise(Time=mean(Time)),aes(xintercept=Time),color='red')+
theme_bw()+
annotate(geom='text',
x=df%>% summarise(Time=mean(Time)) %>% pull(Time)+3,
label=paste0('Avg is ',df%>% summarise(Time=mean(Time)) %>% pull(Time)),
y=7,fontface='bold',size=3)+
theme(axis.text = element_text(color='black',face='bold'),
axis.title = element_text(color='black',face='bold'),
panel.grid = element_blank())
Output:
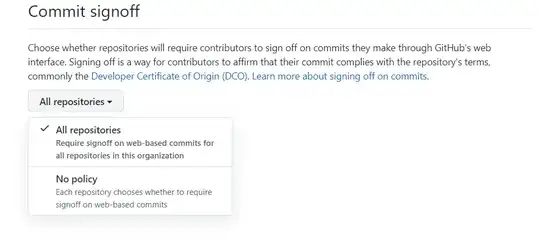
To remove y-axis try this:
#Plot 3
df %>%
arrange(Time) %>%
group_by(Time) %>%
mutate(y=row_number()) %>%
ggplot(aes(x=Time))+
geom_text(aes(y=y,label=ID),size=3,fontface='bold')+
geom_vline(data=df%>% summarise(Time=mean(Time)),aes(xintercept=Time),color='red')+
theme_bw()+
annotate(geom='text',
x=df%>% summarise(Time=mean(Time)) %>% pull(Time)+3,
label=paste0('Avg is ',df%>% summarise(Time=mean(Time)) %>% pull(Time)),
y=7,fontface='bold',size=3)+
theme(axis.text = element_text(color='black',face='bold'),
axis.title = element_text(color='black',face='bold'),
panel.grid = element_blank(),
axis.text.y = element_blank(),
axis.ticks.y = element_blank())+ylab('')
Output:
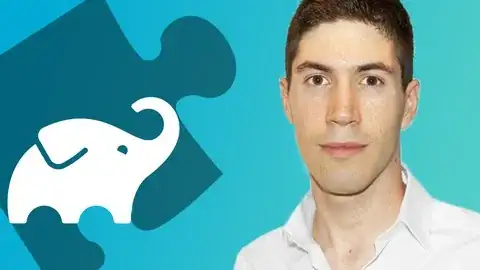