I start by creating mock data in a file called data.txt
with the format you specified:
import numpy as np
def your_func(x, a):
return np.sin(x + a)
n_cycles = 4
n_frames = 100
xmin = 0
xmax = n_cycles*2*np.pi
N = 120
x = np.linspace(xmin, xmax, N)
with open('data.txt', 'w') as fout:
for i in range(n_frames):
alpha = 2*np.pi*i/n_frames
y = your_func(x, alpha)
for value in y:
print(f'{value:.3f}', file=fout)
print(file=fout)
The data file looks like this:
0.000 # First frame
0.210
...
-0.210
-0.000
0.063 # Second frame
0.271
...
-0.148
0.063
...
-0.063 # Frame 100
0.148
...
-0.271
-0.063
Then I read those data from disk:
def read_data(filename):
with open(filename, 'r') as fin:
text = fin.read()
values = [[]]
for line in text.split('\n'):
if line:
values[-1].append(float(line))
else:
values.append([])
return np.array(values[:-2])
y = read_data('data.txt')
The animation is generated as follows:
from matplotlib import pyplot as plt
from matplotlib.animation import FuncAnimation
def plot_background():
line.set_data([], [])
return line,
def animate(i):
line.set_data(x, y[i])
return line,
fig = plt.figure()
ax = plt.axes(xlim=(x[0], x[-1]), ylim=(-1.1, 1.1))
line, = ax.plot([], [], lw=2)
anim = FuncAnimation(fig, animate, init_func=plot_background,
frames=n_frames, interval=10, blit=True)
anim.save(r'animated_function.mp4',
fps=30, extra_args=['-vcodec', 'libx264'])
plt.show()
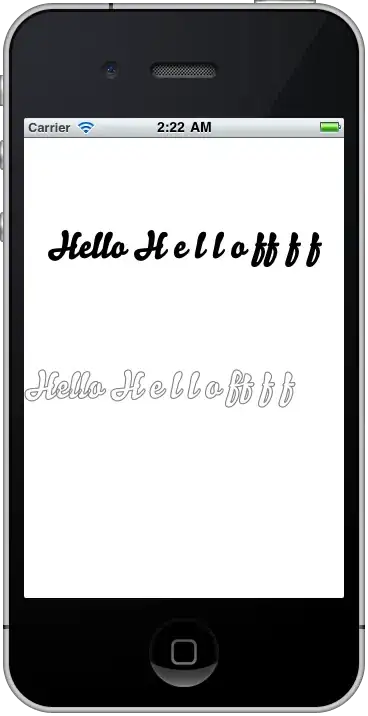
For a more detailed explanation, take a look at this blog.