It's a bad practice to use global
in your code. Instead, you can create a custom attribute for your turtles.
It's super easy, barely an inconvenience:
from turtle import Turtle
pen = Turtle()
pen.lives = 5 # Here, the lives attribute is created
You may even do the same for the font, though it may be unnecessary:
pen.font = ("Arial", 30, "normal")
If loosing lives is the only situation where the life count will be updated, do not keep rewriting them in the loop
(unless, of course, the something gets in the way of the text, and you want the text to be displayed on top),
only rewrite it when a life is lost.
We can redraw and update the lives in a function like this:
def update_lives():
pen.clear()
if pen.lives:
pen.write(f"Lives: {pen.lives}", font=pen.font)
else:
pen.write("You have lost all lives. Try again.", font=pen.font)
pen.lives -= 1 # Remove this line and this becomes your typical text updater
To see this in action, I implemented a demo where a life is lost whenever the user presses the SPACE bar:
from turtle import Turtle, Screen
wn = Screen()
def update_lives():
pen.clear()
if pen.lives:
pen.write(f"Lives: {pen.lives}", font=pen.font)
else:
pen.write("You have lost all lives. Try again.", font=pen.font)
pen.lives -= 1
pen = Turtle(visible=False)
pen.penup()
pen.goto(-300, 200)
pen.lives = 5
pen.font = ("Arial", 30, "normal")
update_lives()
wn.listen()
wn.onkey(update_lives, 'space')
With the above code, when the user reaches 0
, pressing SPACE again will make the function proceed to display negative values.
To fix that, for your main game loop, use while pen.lives
to tell python to only keep looping until the number of lives remaining is greater than 0
:
while pen.lives:
# Your game code here
wn.update()
Output:
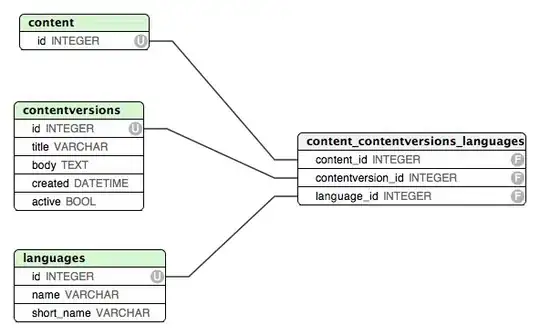