This can be done with the pandas bar plot function. Note that if there are dates that are not recorded in your dataset (e.g. weekends or national holidays) they will not be automatically displayed with a gap in the bar plot. This is because bar plots in pandas (and other packages) are made primarily for categorical data, as mentioned here and here.
import numpy as np # v 1.19.2
import pandas as pd # v 1.1.3
import matplotlib.pyplot as plt # v 3.3.2
# Create a random time series with the date as index
# In your case where you are importing your dataset from excel you
# would assign your date column to the df index like this:
rng = np.random.default_rng(123)
days = 7
df = pd.DataFrame(dict(demand = rng.uniform(100, size=days),
stock = rng.uniform(100, size=days),
origin = np.random.choice(list('ABCD'), days)),
index = pd.date_range(start='2020-12-14', freq='D', periods=days))
# Create pandas bar plot
fig, ax = plt.subplots(figsize=(10,5))
df.plot.bar(ax=ax, color=['tab:blue', 'tab:orange'])
# Assign ticks with custom tick labels
# Date format codes for xticklabels:
# https://docs.python.org/3/library/datetime.html#strftime-and-strptime-format-codes
plt.xticks(ax.get_xticks(), [ts.strftime('%A') for ts in df.index], rotation=0)
plt.legend(frameon=False)
plt.show()
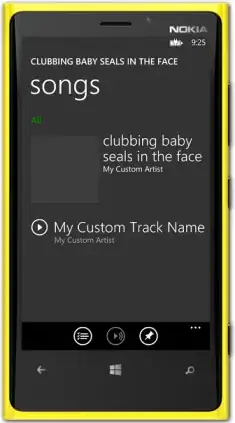