One alternative would be to define symbols for conditional compilation within the project properties.
For instance, you could add, DevEnv17 for VS2022 and DevEnv16 for VS2019.
Ensure you define the right symbols in project properties
<Choose>
<When Condition=" '$(VisualStudioVersion)' == '17.0' ">
<PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Debug|Release|AnyCPU' ">
<DefineConstants>DevEnv17</DefineConstants>
</PropertyGroup>
</When>
<When Condition=" '$(VisualStudioVersion)' == '16.0' ">
<PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Debug|Release|AnyCPU' ">
<DefineConstants>DevEnv16</DefineConstants>
</PropertyGroup>
</When>
</Choose>
Then you could use C# preprocessor directives as below
#if DevEnv17
//Do VS2022 specific stuff
#elif DevEnv16
//Do VS2019 specific stuff
#else
//Do other VS IDE version stuff
#endif
Visual Studio 2022:
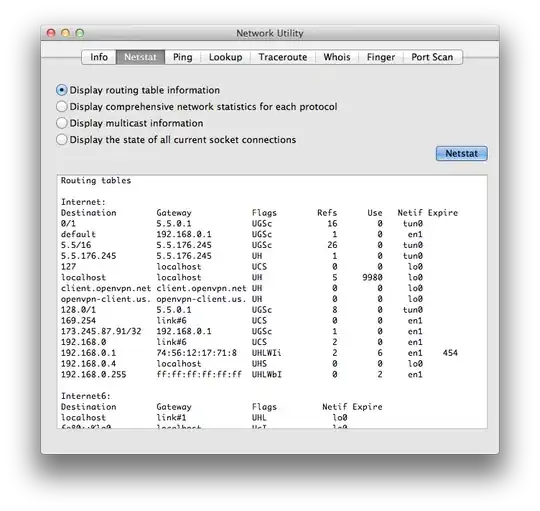
Visual Studio 2019:
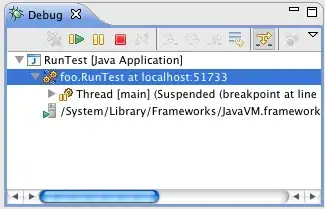